Nuxt Content 3 migration #12
76
.eleventy.js
@ -1,76 +0,0 @@
|
|||||||
const syntaxHighlight = require('@11ty/eleventy-plugin-syntaxhighlight')
|
|
||||||
const markdownIt = require('markdown-it')
|
|
||||||
const markdownItAnchor = require('markdown-it-anchor')
|
|
||||||
const htmlmin = require("html-minifier")
|
|
||||||
|
|
||||||
module.exports = function(eleventyConfig) {
|
|
||||||
// Plugins
|
|
||||||
eleventyConfig.addPlugin(syntaxHighlight)
|
|
||||||
eleventyConfig.addTransform("htmlmin", (content, outputPath) => {
|
|
||||||
if (outputPath.endsWith(".html")) {
|
|
||||||
return htmlmin.minify(content, {
|
|
||||||
collapseWhitespace: true,
|
|
||||||
removeComments: true,
|
|
||||||
useShortDoctype: true,
|
|
||||||
});
|
|
||||||
}
|
|
||||||
|
|
||||||
return content;
|
|
||||||
});
|
|
||||||
|
|
||||||
// To enable merging of tags
|
|
||||||
eleventyConfig.setDataDeepMerge(true)
|
|
||||||
|
|
||||||
// Copy these static files to _site folder
|
|
||||||
eleventyConfig.addPassthroughCopy('src/assets')
|
|
||||||
eleventyConfig.addPassthroughCopy('src/manifest.json')
|
|
||||||
|
|
||||||
// To create excerpts
|
|
||||||
eleventyConfig.setFrontMatterParsingOptions({
|
|
||||||
excerpt: true,
|
|
||||||
excerpt_alias: 'post_excerpt',
|
|
||||||
excerpt_separator: '<!-- excerpt -->'
|
|
||||||
})
|
|
||||||
|
|
||||||
// To create a filter to determine duration of post
|
|
||||||
eleventyConfig.addFilter('readTime', (value) => {
|
|
||||||
const content = value
|
|
||||||
const textOnly = content.split(" ") // content.replace(/(<([^>]+)>)/gi, '')
|
|
||||||
const readingSpeedPerMin = 200
|
|
||||||
return Math.max(1, Math.floor(textOnly.length / readingSpeedPerMin))
|
|
||||||
})
|
|
||||||
|
|
||||||
// get proper date in utc
|
|
||||||
eleventyConfig.addFilter('realDate', (value) => {
|
|
||||||
const actualDate = new Date(value);
|
|
||||||
actualDate.setDate(actualDate.getDate() + 1);
|
|
||||||
return actualDate;
|
|
||||||
})
|
|
||||||
|
|
||||||
// Enable us to iterate over all the tags, excluding posts and all
|
|
||||||
eleventyConfig.addCollection('tagList', collection => {
|
|
||||||
const tagsSet = new Set()
|
|
||||||
collection.getAll().forEach(item => {
|
|
||||||
if (!item.data.tags) return
|
|
||||||
item.data.tags
|
|
||||||
.filter(tag => !['posts', 'all'].includes(tag))
|
|
||||||
.forEach(tag => tagsSet.add(tag))
|
|
||||||
})
|
|
||||||
return Array.from(tagsSet).sort()
|
|
||||||
})
|
|
||||||
|
|
||||||
const md = markdownIt({ linkify: true, html: true })
|
|
||||||
md.use(markdownItAnchor, { level: [1, 2], permalink: true, permalinkBefore: false, permalinkSymbol: '#' })
|
|
||||||
eleventyConfig.setLibrary('md', md)
|
|
||||||
|
|
||||||
// asset_img shortcode
|
|
||||||
eleventyConfig.addLiquidShortcode('asset_img', (filename, css) => {
|
|
||||||
return `<img class="my-4" src="/assets/img/posts/${filename}" style="${css}" />`
|
|
||||||
})
|
|
||||||
|
|
||||||
return {
|
|
||||||
dir: {
|
|
||||||
input: 'src'
|
|
||||||
}
|
|
||||||
}
|
|
||||||
}
|
|
@ -1,2 +0,0 @@
|
|||||||
_site/*
|
|
||||||
!.eleventy.js
|
|
@ -1,34 +0,0 @@
|
|||||||
{
|
|
||||||
"env": {
|
|
||||||
"browser": true,
|
|
||||||
"commonjs": true,
|
|
||||||
"es2020": true,
|
|
||||||
"node": true
|
|
||||||
},
|
|
||||||
"extends": "eslint:recommended",
|
|
||||||
"parserOptions": {
|
|
||||||
"ecmaVersion": 11
|
|
||||||
},
|
|
||||||
"rules": {
|
|
||||||
"indent": [
|
|
||||||
"error",
|
|
||||||
2
|
|
||||||
],
|
|
||||||
"linebreak-style": [
|
|
||||||
"error",
|
|
||||||
"unix"
|
|
||||||
],
|
|
||||||
"quotes": [
|
|
||||||
"error",
|
|
||||||
"single"
|
|
||||||
],
|
|
||||||
"semi": [
|
|
||||||
"error",
|
|
||||||
"never"
|
|
||||||
],
|
|
||||||
"array-element-newline": ["error", {
|
|
||||||
"ArrayExpression": "consistent",
|
|
||||||
"ArrayPattern": { "minItems": 3 }
|
|
||||||
}]
|
|
||||||
}
|
|
||||||
}
|
|
2
.gitattributes
vendored
Normal file
@ -0,0 +1,2 @@
|
|||||||
|
yarn.lock binary
|
||||||
|
package-lock.json binary
|
9
.gitignore
vendored
@ -1,3 +1,8 @@
|
|||||||
package-lock.json
|
|
||||||
_site
|
|
||||||
node_modules
|
node_modules
|
||||||
|
*.log*
|
||||||
|
.nuxt
|
||||||
|
.nitro
|
||||||
|
.cache
|
||||||
|
.output
|
||||||
|
.env
|
||||||
|
dist
|
||||||
|
3
.nuxtignore
Normal file
@ -0,0 +1,3 @@
|
|||||||
|
pages/ignore/*.vue
|
||||||
|
|
||||||
|
layouts/*-ignore.vue
|
43
README.md
@ -1,3 +1,42 @@
|
|||||||
# public
|
# Nuxt 3 Minimal Starter
|
||||||
|
|
||||||
Site at [https://eggworld.tk](https://eggworld.tk)
|
Look at the [nuxt 3 documentation](https://v3.nuxtjs.org) to learn more.
|
||||||
|
|
||||||
|
## Setup
|
||||||
|
|
||||||
|
Make sure to install the dependencies:
|
||||||
|
|
||||||
|
```bash
|
||||||
|
# yarn
|
||||||
|
yarn install
|
||||||
|
|
||||||
|
# npm
|
||||||
|
npm install
|
||||||
|
|
||||||
|
# pnpm
|
||||||
|
pnpm install --shamefully-hoist
|
||||||
|
```
|
||||||
|
|
||||||
|
## Development Server
|
||||||
|
|
||||||
|
Start the development server on http://localhost:3000
|
||||||
|
|
||||||
|
```bash
|
||||||
|
npm run dev
|
||||||
|
```
|
||||||
|
|
||||||
|
## Production
|
||||||
|
|
||||||
|
Build the application for production:
|
||||||
|
|
||||||
|
```bash
|
||||||
|
npm run build
|
||||||
|
```
|
||||||
|
|
||||||
|
Locally preview production build:
|
||||||
|
|
||||||
|
```bash
|
||||||
|
npm run preview
|
||||||
|
```
|
||||||
|
|
||||||
|
Checkout the [deployment documentation](https://v3.nuxtjs.org/guide/deploy/presets) for more information.
|
||||||
|
7
app.vue
Normal file
@ -0,0 +1,7 @@
|
|||||||
|
<template>
|
||||||
|
<div>
|
||||||
|
<NuxtLayout>
|
||||||
|
<NuxtPage />
|
||||||
|
</NuxtLayout>
|
||||||
|
</div>
|
||||||
|
</template>
|
BIN
blog-dark.png
Before Width: | Height: | Size: 120 KiB |
BIN
blog-v2.png
Before Width: | Height: | Size: 117 KiB |
23
create
@ -1,23 +0,0 @@
|
|||||||
#/bin/bash
|
|
||||||
|
|
||||||
TITLE=$@
|
|
||||||
TITLEF=$(echo $TITLE | tr " " "-")
|
|
||||||
YEAR=$(date +"%Y")
|
|
||||||
MONTH=$(date +"%m")
|
|
||||||
|
|
||||||
mkdir -p "src/posts/$YEAR/$MONTH"
|
|
||||||
FILENAME="src/posts/$YEAR/$MONTH/$TITLEF.md"
|
|
||||||
|
|
||||||
cat <<EOF > $FILENAME
|
|
||||||
---
|
|
||||||
title: "$TITLE"
|
|
||||||
date: "$(date '+%Y-%m-%d')"
|
|
||||||
tags:
|
|
||||||
-
|
|
||||||
---
|
|
||||||
|
|
||||||
<!-- excerpt -->
|
|
||||||
|
|
||||||
EOF
|
|
||||||
|
|
||||||
echo "Created new post at $FILENAME"
|
|
6
layouts/default.vue
Normal file
@ -0,0 +1,6 @@
|
|||||||
|
<template>
|
||||||
|
<div>
|
||||||
|
DEFAULT TEMPLATE
|
||||||
|
<slot />
|
||||||
|
</div>
|
||||||
|
</template>
|
15
nuxt.config.ts
Normal file
@ -0,0 +1,15 @@
|
|||||||
|
import { defineNuxtConfig } from "nuxt";
|
||||||
|
|
||||||
|
// https://v3.nuxtjs.org/api/configuration/nuxt.config
|
||||||
|
export default defineNuxtConfig({
|
||||||
|
modules: ["@nuxt/content", "@nuxtjs/tailwindcss"],
|
||||||
|
nitro: {
|
||||||
|
prerender: {
|
||||||
|
routes: ["/sitemap.xml"],
|
||||||
|
},
|
||||||
|
},
|
||||||
|
typescript: {
|
||||||
|
shim: false,
|
||||||
|
},
|
||||||
|
tailwindcss: {},
|
||||||
|
});
|
32
package.json
@ -1,29 +1,15 @@
|
|||||||
{
|
{
|
||||||
"name": "my-blog",
|
"private": true,
|
||||||
"version": "1.0.0",
|
|
||||||
"description": "",
|
|
||||||
"main": "index.js",
|
|
||||||
"scripts": {
|
"scripts": {
|
||||||
"start": "npx @11ty/eleventy --serve & postcss ./tailwind.css --o _site/assets/styles/tailwind.css --watch",
|
"build": "nuxt build",
|
||||||
"test": "echo \"Error: no test specified\" && exit 1",
|
"dev": "nuxt dev",
|
||||||
"lint": "eslint .",
|
"generate": "nuxt generate",
|
||||||
"lint:fix": "eslint . --fix",
|
"preview": "nuxt preview"
|
||||||
"build": "npx @11ty/eleventy & postcss ./tailwind.css --o _site/assets/styles/tailwind.css"
|
|
||||||
},
|
},
|
||||||
"keywords": [],
|
|
||||||
"author": "",
|
|
||||||
"license": "ISC",
|
|
||||||
"devDependencies": {
|
"devDependencies": {
|
||||||
"@11ty/eleventy": ">=0.11.1",
|
"@nuxt/content": "^2.0.1",
|
||||||
"@11ty/eleventy-plugin-syntaxhighlight": ">=3.0.4",
|
"@nuxtjs/tailwindcss": "^5.3.0",
|
||||||
"eslint": ">=7.12.1",
|
"nuxt": "3.0.0-rc.6",
|
||||||
"html-minifier": ">=3.5.21"
|
"sitemap": "^7.1.1"
|
||||||
},
|
|
||||||
"dependencies": {
|
|
||||||
"@tailwindcss/typography": "^0.4.0",
|
|
||||||
"autoprefixer": "^10.2.4",
|
|
||||||
"markdown-it-anchor": "^7.0.1",
|
|
||||||
"postcss-cli": "^8.3.1",
|
|
||||||
"tailwindcss": ">=2.0.2"
|
|
||||||
}
|
}
|
||||||
}
|
}
|
||||||
|
5
pages/[...slug].vue
Normal file
@ -0,0 +1,5 @@
|
|||||||
|
<template>
|
||||||
|
<main>
|
||||||
|
<ContentDoc />
|
||||||
|
</main>
|
||||||
|
</template>
|
@ -1,6 +0,0 @@
|
|||||||
module.exports = {
|
|
||||||
plugins: [
|
|
||||||
require(`tailwindcss`)(`./tailwind.config.js`),
|
|
||||||
require(`autoprefixer`),
|
|
||||||
],
|
|
||||||
};
|
|
@ -1,4 +0,0 @@
|
|||||||
Sitemap: https://eggworld.tk/sitemap.xml
|
|
||||||
|
|
||||||
User-agent: *
|
|
||||||
Disallow:
|
|
11
server/routes/sitemap.xml.ts
Normal file
@ -0,0 +1,11 @@
|
|||||||
|
import { SitemapStream, streamToPromise } from "sitemap";
|
||||||
|
export default defineEventHandler(async (event) => {
|
||||||
|
// Fetch all documents
|
||||||
|
const docs = await serverQueryContent(event).find();
|
||||||
|
const sitemap = new SitemapStream({ hostname: "https://example.com" });
|
||||||
|
for (const doc of docs) {
|
||||||
|
sitemap.write({ url: doc._path, changefreq: "monthly" });
|
||||||
|
}
|
||||||
|
sitemap.end();
|
||||||
|
return streamToPromise(sitemap);
|
||||||
|
});
|
@ -1,7 +0,0 @@
|
|||||||
---
|
|
||||||
layout: default
|
|
||||||
title: Not all who wander are lost
|
|
||||||
---
|
|
||||||
|
|
||||||
The page you are looking for does not seem to exist.
|
|
||||||
But thanks for dropping by.
|
|
@ -1,9 +0,0 @@
|
|||||||
{
|
|
||||||
"name": "Eggy",
|
|
||||||
"url": "https://eggworld.tk",
|
|
||||||
"title": "Eggworld",
|
|
||||||
"description": "Ramblings and ideas",
|
|
||||||
"github": "https://github.com/potatoeggy",
|
|
||||||
"twitter": "https://twitter.com/potatoeggy",
|
|
||||||
"linkedin": "https://linkedin.com/in/potatoeggy"
|
|
||||||
}
|
|
@ -1,7 +0,0 @@
|
|||||||
<div class="mt-16 py-8 border-t-2 flex justify-between items-center">
|
|
||||||
<div class="flex items-center flex-1">
|
|
||||||
<div class="inline-block h-8 w-8 bg-pink-400 rounded-full mr-2 flex justify-center items-center">D</div>
|
|
||||||
<span class="text-lg font-medium">{{ site.name }}</span>
|
|
||||||
</div>
|
|
||||||
<p class="text-sm flex-1">Student and Linux enthusiast. Likes poutine.</p>
|
|
||||||
</div>
|
|
@ -1,43 +0,0 @@
|
|||||||
<!doctype html>
|
|
||||||
<html lang="en">
|
|
||||||
<head>
|
|
||||||
<meta charset="utf-8">
|
|
||||||
<meta name='viewport' content='width=device-width'>
|
|
||||||
|
|
||||||
<title>{{ title }} | {{ site.title }}</title>
|
|
||||||
<meta name="description" content="{% if post_excerpt %}{{ post_excerpt | escape }}{% else %}{{ site.description }}{% endif %}">
|
|
||||||
<meta name="theme-color" content="#ffffff"/>
|
|
||||||
|
|
||||||
<link rel="icon" type="image/png" sizes="32x32" href="/assets/img/favicon/favicon-32x32.png">
|
|
||||||
<link rel="apple-touch-icon" href="/assets/img/favicon/apple-touch-icon.png">
|
|
||||||
<link rel="manifest" href="/manifest.json" />
|
|
||||||
|
|
||||||
<link href="https://unpkg.com/prismjs@1.20.0/themes/prism-okaidia.css" rel="stylesheet">
|
|
||||||
<link href="/assets/styles/tailwind.css" rel="stylesheet" />
|
|
||||||
<link href="/assets/styles/index.css" rel="stylesheet" />
|
|
||||||
<script>
|
|
||||||
const isDarkMode = () => localStorage.theme === 'dark' || (!('theme' in localStorage) && window.matchMedia('(prefers-color-scheme: dark)').matches);
|
|
||||||
if (isDarkMode()) {
|
|
||||||
document.documentElement.classList.add('dark')
|
|
||||||
} else {
|
|
||||||
document.documentElement.classList.remove('dark')
|
|
||||||
}
|
|
||||||
</script>
|
|
||||||
</head>
|
|
||||||
|
|
||||||
<body class="dark:text-white dark:bg-gray-900">
|
|
||||||
{{ content }}
|
|
||||||
|
|
||||||
<script>
|
|
||||||
document.getElementById("toggleDarkMode").addEventListener("click", function() {
|
|
||||||
if (isDarkMode()) {
|
|
||||||
localStorage.theme = 'light'
|
|
||||||
document.documentElement.classList.remove('dark')
|
|
||||||
} else {
|
|
||||||
localStorage.theme = 'dark'
|
|
||||||
document.documentElement.classList.add('dark')
|
|
||||||
}
|
|
||||||
});
|
|
||||||
</script>
|
|
||||||
</body>
|
|
||||||
</html>
|
|
@ -1,11 +0,0 @@
|
|||||||
---
|
|
||||||
layout: main
|
|
||||||
---
|
|
||||||
|
|
||||||
<p class="text-5xl md:text-5xl font-black py-10 md:py-5 leading-tight">
|
|
||||||
{{ title }}
|
|
||||||
</p>
|
|
||||||
|
|
||||||
{% include nav %}
|
|
||||||
|
|
||||||
{{ content }}
|
|
@ -1,17 +0,0 @@
|
|||||||
<footer class="mt-8 bg-gray-200 dark:bg-black py-8 flex-shrink-0">
|
|
||||||
<div class="max-w-screen-sm md:max-w-screen-md mx-auto px-8">
|
|
||||||
<p class="text-sm py-2 text-right">
|
|
||||||
{% if site.github != "" %}
|
|
||||||
<a href={{ site.github }}>Github</a>
|
|
||||||
{% endif %}
|
|
||||||
|
|
||||||
{% if site.linkedin != "" %}
|
|
||||||
· <a href={{ site.linkedin }}>LinkedIn</a>
|
|
||||||
{% endif %}
|
|
||||||
|
|
||||||
{% if site.twitter != "" %}
|
|
||||||
· <a href={{ site.twitter }}>Twitter</a>
|
|
||||||
{% endif %}
|
|
||||||
</p>
|
|
||||||
</div>
|
|
||||||
</footer>
|
|
@ -1,14 +0,0 @@
|
|||||||
---
|
|
||||||
layout: base
|
|
||||||
---
|
|
||||||
|
|
||||||
<div class="min-h-screen flex flex-col">
|
|
||||||
|
|
||||||
<main class="flex-1 w-10/12 max-w-screen-sm md:max-w-screen-md mx-auto">
|
|
||||||
|
|
||||||
{{ content }}
|
|
||||||
|
|
||||||
</main>
|
|
||||||
|
|
||||||
{% include footer %}
|
|
||||||
</div>
|
|
@ -1,9 +0,0 @@
|
|||||||
<nav>
|
|
||||||
<ul class="flex py-2 border-b-2 mb-6">
|
|
||||||
<li class="mr-6"><a href="/">all</a></li>
|
|
||||||
<li class="mr-6"><a href="/tags">tags</a></li>
|
|
||||||
<li class="mr-6"><a href="/about">about</a></li>
|
|
||||||
<li class="mr-6"><a href="/services">services</a></li>
|
|
||||||
<li class="mr-6"><span class="cursor-pointer" id="toggleDarkMode">dark</span></li>
|
|
||||||
</ul>
|
|
||||||
</nav>
|
|
@ -1,72 +0,0 @@
|
|||||||
<nav class="flex justify-center mt-8">
|
|
||||||
<div class="inline-flex">
|
|
||||||
{% if page.url != pagination.href.first %}
|
|
||||||
<a href="{{ pagination.href.first }}">
|
|
||||||
<button class="paginator-text">
|
|
||||||
First
|
|
||||||
</button>
|
|
||||||
</a>
|
|
||||||
{% else %}
|
|
||||||
<button class="paginator-text cursor-not-allowed">
|
|
||||||
First
|
|
||||||
</button>
|
|
||||||
{% endif %}
|
|
||||||
|
|
||||||
|
|
||||||
{% if pagination.href.previous %}
|
|
||||||
<a href="{{ pagination.href.previous }}">
|
|
||||||
<button class="py-2 px-4">
|
|
||||||
<img src="/assets/img/chevron-left.svg" alt="prev">
|
|
||||||
</button>
|
|
||||||
</a>
|
|
||||||
{% else %}
|
|
||||||
<button class="py-2 px-4 cursor-not-allowed">
|
|
||||||
<img src="/assets/img/chevron-left.svg" alt="prev">
|
|
||||||
</button>
|
|
||||||
{% endif %}
|
|
||||||
|
|
||||||
{% for pageEntry in pagination.pages %}
|
|
||||||
{% if page.url == pagination.hrefs[forloop.index0] %}
|
|
||||||
<a href="{{ pagination.hrefs[forloop.index0] }}" aria-current="page">
|
|
||||||
<button class="border bg-gray-300 hover:bg-gray-400 text-gray-800 font-bold py-2 px-4">
|
|
||||||
{{ forloop.index }}
|
|
||||||
</button>
|
|
||||||
</a>
|
|
||||||
{% else %}
|
|
||||||
<a href="{{ pagination.hrefs[forloop.index0] }}">
|
|
||||||
<button class="border border-gray-300 bg-white hover:bg-gray-300 text-gray-800 font-bold py-2 px-4">
|
|
||||||
{{ forloop.index }}
|
|
||||||
</button>
|
|
||||||
</a>
|
|
||||||
{% endif %}
|
|
||||||
{% endfor %}
|
|
||||||
|
|
||||||
|
|
||||||
{% if pagination.href.next %}
|
|
||||||
<a href="{{ pagination.href.next }}">
|
|
||||||
<button class="py-2 px-4">
|
|
||||||
<img src="/assets/img/chevron-right.svg" alt="next">
|
|
||||||
</button>
|
|
||||||
</a>
|
|
||||||
{% else %}
|
|
||||||
<button class="py-2 px-4 cursor-not-allowed">
|
|
||||||
<img src="/assets/img/chevron-right.svg" alt="next">
|
|
||||||
</button>
|
|
||||||
{% endif %}
|
|
||||||
|
|
||||||
|
|
||||||
{% if page.url != pagination.href.last %}
|
|
||||||
<a href="{{ pagination.href.last }}">
|
|
||||||
<button class="paginator-text">
|
|
||||||
Last
|
|
||||||
</button>
|
|
||||||
</a>
|
|
||||||
{% else %}
|
|
||||||
<button class="paginator-text cursor-not-allowed">
|
|
||||||
Last
|
|
||||||
</button>
|
|
||||||
{% endif %}
|
|
||||||
|
|
||||||
</div>
|
|
||||||
|
|
||||||
</nav>
|
|
@ -1,30 +0,0 @@
|
|||||||
---
|
|
||||||
layout: main
|
|
||||||
---
|
|
||||||
|
|
||||||
{% include nav %}
|
|
||||||
|
|
||||||
<p class="text-5xl md:text-4xl font-black pt-10 md:pt-30 pb-4 leading-tight">
|
|
||||||
{{ title }}
|
|
||||||
</p>
|
|
||||||
|
|
||||||
<div>
|
|
||||||
<em>{{ date | realDate | date: "%Y-%m-%d" }}</em> ·
|
|
||||||
<span>{{ content | readTime }} min read</span>
|
|
||||||
</div>
|
|
||||||
|
|
||||||
<div class="mt-3 pb-10">
|
|
||||||
{% for tag in tags %}
|
|
||||||
{% if tag != "posts" %}
|
|
||||||
<a href="/tags/{{ tag }}">
|
|
||||||
<span class="post-tag">{{ tag }}</span>
|
|
||||||
</a>
|
|
||||||
{% endif %}
|
|
||||||
{% endfor %}
|
|
||||||
</div>
|
|
||||||
|
|
||||||
<div class="prose dark:prose-dark">
|
|
||||||
{{ content }}
|
|
||||||
</div>
|
|
||||||
|
|
||||||
{% include author %}
|
|
@ -1,7 +0,0 @@
|
|||||||
---
|
|
||||||
layout: default
|
|
||||||
---
|
|
||||||
|
|
||||||
<div class="prose dark:prose-dark">
|
|
||||||
{{ content }}
|
|
||||||
</div>
|
|
20
src/about.md
@ -1,20 +0,0 @@
|
|||||||
---
|
|
||||||
layout: typical
|
|
||||||
title: About
|
|
||||||
---
|
|
||||||
|
|
||||||
Hello! It's very nice to meet you — I'm a student and Linux enthusiast who is quite passionate about some subjects but is quite lazy in every other.
|
|
||||||
|
|
||||||
I've dabbled extensively and non-extensively in a variety of topics to play with, including:
|
|
||||||
|
|
||||||
- competitive programming on [DMOJ](https://dmoj.ca/user/eggy)
|
|
||||||
- GUI toolkits very very briefly in GTK, Qt, and Swing
|
|
||||||
- Linux and server administration
|
|
||||||
- web "development" in the form of a Chrome extension and my sites
|
|
||||||
- Godot Engine Cat Simulator DX
|
|
||||||
- ski instruction
|
|
||||||
- writing of literature
|
|
||||||
- emulation
|
|
||||||
- Discord bots
|
|
||||||
|
|
||||||
…and other things that I'm forgetting right now.
|
|
@ -1,4 +0,0 @@
|
|||||||
<svg xmlns="http://www.w3.org/2000/svg" class="icon icon-tabler icon-tabler-chevron-left" width="24" height="24" viewBox="0 0 24 24" stroke-width="1.5" stroke="#000000" fill="none" stroke-linecap="round" stroke-linejoin="round">
|
|
||||||
<path stroke="none" d="M0 0h24v24H0z"/>
|
|
||||||
<polyline points="15 6 9 12 15 18" />
|
|
||||||
</svg>
|
|
Before Width: | Height: | Size: 317 B |
@ -1,4 +0,0 @@
|
|||||||
<svg xmlns="http://www.w3.org/2000/svg" class="icon icon-tabler icon-tabler-chevron-right" width="24" height="24" viewBox="0 0 24 24" stroke-width="1.5" stroke="#000000" fill="none" stroke-linecap="round" stroke-linejoin="round">
|
|
||||||
<path stroke="none" d="M0 0h24v24H0z"/>
|
|
||||||
<polyline points="9 6 15 12 9 18" />
|
|
||||||
</svg>
|
|
Before Width: | Height: | Size: 317 B |
@ -1,5 +0,0 @@
|
|||||||
<svg xmlns="http://www.w3.org/2000/svg" class="icon icon-tabler icon-tabler-chevrons-left" width="24" height="24" viewBox="0 0 24 24" stroke-width="1.5" stroke="#000000" fill="none" stroke-linecap="round" stroke-linejoin="round">
|
|
||||||
<path stroke="none" d="M0 0h24v24H0z"/>
|
|
||||||
<polyline points="11 7 6 12 11 17" />
|
|
||||||
<polyline points="17 7 12 12 17 17" />
|
|
||||||
</svg>
|
|
Before Width: | Height: | Size: 359 B |
Before Width: | Height: | Size: 7.9 KiB |
Before Width: | Height: | Size: 8.6 KiB |
Before Width: | Height: | Size: 1.2 KiB |
Before Width: | Height: | Size: 30 KiB |
Before Width: | Height: | Size: 88 KiB |
Before Width: | Height: | Size: 310 KiB |
Before Width: | Height: | Size: 143 KiB |
Before Width: | Height: | Size: 144 KiB |
Before Width: | Height: | Size: 49 KiB |
Before Width: | Height: | Size: 788 KiB |
Before Width: | Height: | Size: 8.4 KiB |
Before Width: | Height: | Size: 855 KiB |
Before Width: | Height: | Size: 966 KiB |
Before Width: | Height: | Size: 907 KiB |
Before Width: | Height: | Size: 494 KiB |
Before Width: | Height: | Size: 1.9 MiB |
Before Width: | Height: | Size: 1.2 MiB |
Before Width: | Height: | Size: 52 KiB |
Before Width: | Height: | Size: 71 KiB |
@ -1,4 +0,0 @@
|
|||||||
.header-anchor {
|
|
||||||
color: grey!important;
|
|
||||||
text-decoration: none!important;
|
|
||||||
}
|
|
@ -1,41 +0,0 @@
|
|||||||
---
|
|
||||||
layout: default
|
|
||||||
title: All
|
|
||||||
pagination:
|
|
||||||
data: collections.posts
|
|
||||||
size: 50
|
|
||||||
reverse: true
|
|
||||||
alias: posts
|
|
||||||
---
|
|
||||||
|
|
||||||
{% for post in pagination.items %}
|
|
||||||
{% if post.data.published %}
|
|
||||||
<div class="py-4">
|
|
||||||
<p>
|
|
||||||
<span class="text-2xl sm:text-2xl font-bold hover:text-blue-700 leading-tight"><a href="{{ post.url }}">{{ post.data.title }}</a></span>
|
|
||||||
<span class="text-base sm:text-2xs font-normal"> · {{ post.templateContent | readTime }} min read</span>
|
|
||||||
</p>
|
|
||||||
<em>{{ post.date | realDate | date: "%Y-%m-%d" }}</em>
|
|
||||||
<p class="mt-4">{{ post.data.post_excerpt }}...</p>
|
|
||||||
|
|
||||||
<div class="flex justify-between items-center mt-4">
|
|
||||||
<div class="flex-1 pr-4">
|
|
||||||
{% for tag in post.data.tags %}
|
|
||||||
{% if tag != "posts" %}
|
|
||||||
<a href="/tags/{{ tag }}" class="">
|
|
||||||
<div class="post-tag">{{ tag }}</div>
|
|
||||||
</a>
|
|
||||||
{% endif %}
|
|
||||||
{% endfor %}
|
|
||||||
</div>
|
|
||||||
|
|
||||||
<a class="flex-none hover:underline font-semibold text-blue-700" href="{{ post.url }}">Read this post →</a>
|
|
||||||
</div>
|
|
||||||
|
|
||||||
</div>
|
|
||||||
|
|
||||||
{% endif %}
|
|
||||||
{% endfor %}
|
|
||||||
|
|
||||||
|
|
||||||
{% include paginator %}
|
|
@ -1,26 +0,0 @@
|
|||||||
{
|
|
||||||
"name": "Eggworld",
|
|
||||||
"short_name": "Eggworld",
|
|
||||||
"icons": [
|
|
||||||
{
|
|
||||||
"src": "/assets/img/favicon/favicon-32x32.png",
|
|
||||||
"sizes": "32x32",
|
|
||||||
"type": "image/png",
|
|
||||||
"purpose": "any maskable"
|
|
||||||
},
|
|
||||||
{
|
|
||||||
"src": "/assets/img/favicon/favicon-192x192.png",
|
|
||||||
"sizes": "192x192",
|
|
||||||
"type": "image/png"
|
|
||||||
},
|
|
||||||
{
|
|
||||||
"src": "/assets/img/favicon/favicon-512x512.png",
|
|
||||||
"sizes": "512x512",
|
|
||||||
"type": "image/png"
|
|
||||||
}
|
|
||||||
],
|
|
||||||
"theme_color": "#000",
|
|
||||||
"background_color": "#fff",
|
|
||||||
"display": "standalone",
|
|
||||||
"start_url": "/"
|
|
||||||
}
|
|
@ -1,13 +0,0 @@
|
|||||||
---
|
|
||||||
title: On the Topic of Dark Mode
|
|
||||||
date: 2021-04-07
|
|
||||||
tags:
|
|
||||||
- blog
|
|
||||||
- misc
|
|
||||||
---
|
|
||||||
On the desktop, dark mode is an abomination that should be eradicated from applications.
|
|
||||||
<!-- excerpt -->
|
|
||||||
|
|
||||||
Browsers, IDEs, and other applications must be freed from their shadowy chains and returned to light — where they truly belong.
|
|
||||||
|
|
||||||
{% asset_img "light-discord.png" "Perfect." %}
|
|
@ -1,41 +0,0 @@
|
|||||||
---
|
|
||||||
title: An Introduction to the Objective-Subjective Scoring System
|
|
||||||
date: 2021-04-14
|
|
||||||
tags:
|
|
||||||
- blog
|
|
||||||
- misc
|
|
||||||
---
|
|
||||||
|
|
||||||
It's difficult to fairly judge anything in a manner free from bias. It's even more difficult to do it for abstract and creative works such as literature or stories, as your own personal enjoyment can greatly affect how you view the work and any flaws it might have. The **Objective-Subjective Scoring System (OS3)** attempts to remedy this issue by separating as much bias as possible.
|
|
||||||
|
|
||||||
<!-- excerpt -->
|
|
||||||
|
|
||||||
The primary feature of OS3 is that it assigns two scores to a certain work with a small scale: the *objective score* and the *subjective score*, both inclusively ranging from 0 to 3.
|
|
||||||
|
|
||||||
## The objective score
|
|
||||||
|
|
||||||
The objective score should be representative of a work's clear quality relative to other works and how "good" it is. It should be as free from bias as possible and should have clear, defined criteria so that the standard can be consistently applied to other works. Ideally, an objective score should not be controversial and others who consume the same work should assign the same score. A sample criteria which I use for stories is provided below:
|
|
||||||
|
|
||||||
- **0:** The story does not meet expectations and contains large character, story, and/or formatting flaws that severely detract from the experience of the story, or worse. The story is *rejected objectively*.
|
|
||||||
- **1:** The story nearly fails to meets expectations and contains large character, story, and/or formatting flaws that severely detract from the experience of the story.
|
|
||||||
- **2:** The story meets expectations but contains flaws that do not severely detract from the. experience of the story.
|
|
||||||
- **3:** The story meets expectations and may contain flaws that do not detract from the experience of the story, or better.
|
|
||||||
|
|
||||||
The objective score is relative. To provide a meaningful score, there are only four options so it is easier to assign a certain score. Most typical stories should fall under an objective one or two. To reduce the influence of opinions on the objective score, it should be determined after the subjective score.
|
|
||||||
|
|
||||||
## The subjective score
|
|
||||||
|
|
||||||
The subjective score is…subjective. It should reflect your personal enjoyment of a work. Similar to the objective score, it should be kept consistent — a work with a subjective three should be without question to be more preferred than one with a subjective two. Clear and defined criteria are helpful here, but not as required as like in determining an objective score. A sample criteria which I use for stories is proided below:
|
|
||||||
|
|
||||||
- **0:** The story is not enjoyable and was dropped before completion. The story is *rejected subjectively*.
|
|
||||||
- **1:** The story is mildly enjoyable and was completed but likely will not be re-consumed very often if at all.
|
|
||||||
- **2:** The story is enjoyable and will be re-consumed from time to time.
|
|
||||||
- **3:** The story is very enjoyable and will most certainly be re-consumed fairly often.
|
|
||||||
|
|
||||||
## Influences and limitations
|
|
||||||
|
|
||||||
The objective of the OS3 is to minimise the impact of the subjective score on the objective score. However, it does nothing to prevent the opposite — which is fine. How "good" a work is likely has a direct impact on your enjoyment of it, anyway. This creates an effect where low objective scores are commonly paired up with low subjective scores, and high objective scores more commonly have high subjective scores.
|
|
||||||
|
|
||||||
Although this system can reduce the influence on bias in determining the quality of a work, it is still ultimately up to you to enforce a strict standard and not award works higher objective scores due to liking them more. I've found myself that I've had to reduce objective scores multiple times typically after re-consuming a work and looking at it from a more critical angle.
|
|
||||||
|
|
||||||
Happy judging!
|
|
@ -1,35 +0,0 @@
|
|||||||
---
|
|
||||||
title: Why Self-Host?
|
|
||||||
date: 2021-04-16
|
|
||||||
tags:
|
|
||||||
- blog
|
|
||||||
- tech
|
|
||||||
---
|
|
||||||
|
|
||||||
There are a variety of services out there that make it easy for anyone to set up their own "cloud". From Google Drive for storage to Medium for blogs, there are unlimited options in putting content out there. So aside as a learning experience, why would you take on the burden of running a server on your own machine?
|
|
||||||
|
|
||||||
<!-- excerpt -->
|
|
||||||
|
|
||||||
## Greater control
|
|
||||||
|
|
||||||
The big reason is to have greater control over your data. For those that are more privacy-inclined, not having to subject their content to service providers' terms and conditions may be a boon. You can control who sees your content, precisely how they see it, and reduce any bloat that providers attach to web pages.
|
|
||||||
|
|
||||||
Additionally, you gain extremely low-level access to the administration of your server, which can be helpful if you like to tinker and fix something yourself or would like to make a deep change in how your server is configured at the OS level.
|
|
||||||
|
|
||||||
You can integrate different services more easily and run them together — for example, Syncthing ensures all of my music files are constantly kept up-to-date and accessible between my laptop, phone, and Plex.
|
|
||||||
|
|
||||||
## Custom services
|
|
||||||
|
|
||||||
On the off chance that you have a niche service that the cloud can't provide to you, you might turn to self-hosting. For example, to keep my Kobo up-to-date with any stories I add, I use Calibre-web combined with Syncthing to automatically download books as I add via my computer. It also provides a nice web interface that I can access if I happen to want to read something but don't have any of my personal devices with me.
|
|
||||||
|
|
||||||
You can also run things that normally you would have to pay a subscription fee for — large file storage and music/video/game streaming with Nextcloud and Plex/Steam respectively are just a couple of examples. Services that also charge extra for custom domain integration can also be avoided by self-hosting instead if you would like to keep it fancy. Game servers and websites come into mind.
|
|
||||||
|
|
||||||
## Limitations
|
|
||||||
|
|
||||||
Although self-hosting is certainly fun, it is subject to several issues that make it impractical for most, aside from the hardware and bandwidth/data cost.
|
|
||||||
|
|
||||||
Fairly advanced technical knowledge is required to set up such a server and maintain it without breakage. Even so, things do happen — typically after system updates — and there is also a massive time investment in a home server. It is also important to be aware of best security practices on the chance that a malicious actor could use your server to infiltrate your network. With paid services, they can largely guarantee that the underlying operating system will not break on you and that security is taken care of. This is amplified as your website or service becomes more popular.
|
|
||||||
|
|
||||||
## Conclusion
|
|
||||||
|
|
||||||
Running your own server is a lot of work. But it puts my old computer to good use, and has personally helped me a lot in learning more about how our world today operates, even if I only get a small glimpse in that world. My Optiplex provides a variety of helpful services that I regularly use and some that I would not be able to obtain otherwise in exchange for a large chunk of my time. Depending on your circumstances, however, you might be better off relying on others instead. It's a good idea to weigh the pros and cons of investing any monetary resources or time into such an idea.
|
|
@ -1,15 +0,0 @@
|
|||||||
---
|
|
||||||
title: Being Complacent
|
|
||||||
date: 2021-05-21
|
|
||||||
tags:
|
|
||||||
- blog
|
|
||||||
- misc
|
|
||||||
---
|
|
||||||
|
|
||||||
Imagine this: you think you're doing great in the world, and all your friends are telling you you're doing great in the world. But one day, you look outside the bubble you're in and realise just how far behind you really are.
|
|
||||||
|
|
||||||
<!-- excerpt -->
|
|
||||||
|
|
||||||
This is what complacency leads to. By limiting your own perspective and telling yourself that you're doing great, you don't notice how everyone else is capable of so much more than you are. Even if you once had a near-insurmountable lead, by no longer applying pressure to keep moving forward, or by reducing pressure to the point that you *feel* like you're moving forward but are practically staying still, anyone can catch up should they decide to.
|
|
||||||
|
|
||||||
It is important to avoid being complacent. Never think what you're doing is completely enough. Continuously push your boundaries. Like in Maoism, "constant revolution" is needed to "prevent counter-revolution". Constant progression is required to prevent stagnation.
|
|
@ -1,262 +0,0 @@
|
|||||||
---
|
|
||||||
title: Handy Python Tips
|
|
||||||
date: 2021-05-29
|
|
||||||
tags:
|
|
||||||
- blog
|
|
||||||
- tech
|
|
||||||
---
|
|
||||||
|
|
||||||
Python is a flexible programming language that is well-known for its simplicity and numerous features that make programming in it a lot faster. This article presents just a few that might speed up your productivity a little bit.
|
|
||||||
|
|
||||||
<!-- excerpt -->
|
|
||||||
|
|
||||||
## 1. Tuple expansion
|
|
||||||
|
|
||||||
```python
|
|
||||||
a, b = tuple([1, 2])
|
|
||||||
s1, s2 = input(), int(input())
|
|
||||||
```
|
|
||||||
|
|
||||||
Tuple expansion is one of Python's most powerful features, allowing you to assign multiple values or expand multiple values of a tuple in a single line.
|
|
||||||
|
|
||||||
```python
|
|
||||||
>>> print(a, b)
|
|
||||||
1 2
|
|
||||||
>>> print(s1, s2)
|
|
||||||
baguette
|
|
||||||
pomme
|
|
||||||
Traceback (most recent call last):
|
|
||||||
File "<stdin>", line 1, in <module>
|
|
||||||
ValueError: invalid literal for int() with base 10: 'pomme'
|
|
||||||
```
|
|
||||||
|
|
||||||
The code above effectively assigns a tuple `(a, b)` to another tuple `(1, 2)`, and is equivalent to the code below:
|
|
||||||
|
|
||||||
```python
|
|
||||||
a = 1
|
|
||||||
b = 2
|
|
||||||
s1 = input()
|
|
||||||
s2 = int(input())
|
|
||||||
```
|
|
||||||
|
|
||||||
This can let you return multiple values from a function.
|
|
||||||
|
|
||||||
```python
|
|
||||||
def init():
|
|
||||||
return (5, 6)
|
|
||||||
|
|
||||||
a, b = init()
|
|
||||||
print(a, b)
|
|
||||||
```
|
|
||||||
|
|
||||||
Output:
|
|
||||||
|
|
||||||
```
|
|
||||||
5 6
|
|
||||||
```
|
|
||||||
|
|
||||||
Tuple expansion is also used in for loops to iterate over tuples or even dictionaries.
|
|
||||||
|
|
||||||
```python
|
|
||||||
array = [
|
|
||||||
(1, 2),
|
|
||||||
(3, 4),
|
|
||||||
(5, 6)
|
|
||||||
]
|
|
||||||
|
|
||||||
items = {
|
|
||||||
7: 8,
|
|
||||||
9: 10,
|
|
||||||
11: 12
|
|
||||||
}
|
|
||||||
|
|
||||||
for i, j in array:
|
|
||||||
print(i, j)
|
|
||||||
|
|
||||||
for k, v in items.items():
|
|
||||||
print(k, v)
|
|
||||||
```
|
|
||||||
|
|
||||||
Output:
|
|
||||||
|
|
||||||
```
|
|
||||||
1 2
|
|
||||||
3 4
|
|
||||||
5 6
|
|
||||||
7 8
|
|
||||||
9 10
|
|
||||||
11 12
|
|
||||||
```
|
|
||||||
|
|
||||||
## 2. Nicer iteration with zip() and enumerate()
|
|
||||||
|
|
||||||
Python's for loop is commonly known in other programming languages as a for-each loop. This is great if you just want each item in an iterable, but sometimes you want the index too! Instead of having to resort to `range(len(array))`, instead you can use `enumerate()` and tuple expansion to easily get both the index of the element and the element itself:
|
|
||||||
|
|
||||||
```python
|
|
||||||
array = ["a", "b", "c", "d", "e"]
|
|
||||||
for i, c in enumerate(array):
|
|
||||||
print(i, c)
|
|
||||||
```
|
|
||||||
|
|
||||||
Output:
|
|
||||||
|
|
||||||
```
|
|
||||||
0 a
|
|
||||||
1 b
|
|
||||||
2 c
|
|
||||||
3 d
|
|
||||||
4 e
|
|
||||||
```
|
|
||||||
|
|
||||||
In a similar vein, if you have two arrays you want to process at the same time, you don't need to use `range(len(array))` when you have `zip()`, which will bundle the different iterators into one big one as big as the smallest iterable.
|
|
||||||
|
|
||||||
```python
|
|
||||||
ints = [1, 2, 3, 4]
|
|
||||||
strs = ("pomme", "poutine", "pinterest", "pear")
|
|
||||||
floats = [1.0, 2.0, 3.0, 4.0, 5.0, 6.0]
|
|
||||||
for i, s, f in zip(ints, strs, floats):
|
|
||||||
print(i, s, f)
|
|
||||||
```
|
|
||||||
|
|
||||||
Output:
|
|
||||||
|
|
||||||
```
|
|
||||||
1 pomme 1.0
|
|
||||||
2 poutine 2.0
|
|
||||||
3 pinterest 3.0
|
|
||||||
4 pear 4.0
|
|
||||||
```
|
|
||||||
|
|
||||||
## 3. Iterable unpacking
|
|
||||||
|
|
||||||
In competitive programming, often you have to print out space-separated results. This can be done by the mildly inconveniencing
|
|
||||||
|
|
||||||
```python
|
|
||||||
print(" ".join([1, 2, 3, 4]))
|
|
||||||
```
|
|
||||||
|
|
||||||
or heaven forbid, via iteration:
|
|
||||||
|
|
||||||
```python
|
|
||||||
for i in [1, 2, 3, 4]:
|
|
||||||
print(i, end=" ")
|
|
||||||
print()
|
|
||||||
```
|
|
||||||
|
|
||||||
which is where iterable unpacking comes in, and you can go straight to
|
|
||||||
|
|
||||||
```python
|
|
||||||
print(*[1, 2, 3, 4])
|
|
||||||
```
|
|
||||||
|
|
||||||
The **unpacking operator** (the asterisk) basically gets rid of the container and throws all of the elements inside directly into the print function as parameters.
|
|
||||||
|
|
||||||
That means that the above line of code is equivalent to:
|
|
||||||
|
|
||||||
```python
|
|
||||||
print(1, 2, 3, 4)
|
|
||||||
```
|
|
||||||
|
|
||||||
which nicely prints out the integers separated by spaces with a newline at the end.
|
|
||||||
|
|
||||||
But wait, there's more! The unpacking operator is also commonly used in function definitions as a catch-all parameter for extra arguments, stuffing them into a list.
|
|
||||||
|
|
||||||
```python
|
|
||||||
def init(a, b, *args):
|
|
||||||
print(a, b)
|
|
||||||
print(args)
|
|
||||||
|
|
||||||
init(1, 2, "pomme", 4, 6.0)
|
|
||||||
```
|
|
||||||
|
|
||||||
Output:
|
|
||||||
|
|
||||||
```
|
|
||||||
1, 2
|
|
||||||
['pomme', 4, 6.0]
|
|
||||||
```
|
|
||||||
|
|
||||||
You can also use this in normal assignment:
|
|
||||||
|
|
||||||
```python
|
|
||||||
a, *args, b = (1, 2, 3, 4, 5)
|
|
||||||
print(a)
|
|
||||||
print(args)
|
|
||||||
print(b)
|
|
||||||
```
|
|
||||||
|
|
||||||
Output:
|
|
||||||
|
|
||||||
```
|
|
||||||
1
|
|
||||||
[2, 3, 4]
|
|
||||||
5
|
|
||||||
```
|
|
||||||
|
|
||||||
And it can be used as a handy way to expand a list instead of using `list()`. The comma at the end of the variable is there to indicate it is a tuple with a single element (a singleton).
|
|
||||||
|
|
||||||
```python
|
|
||||||
*s, = "abcde"
|
|
||||||
print(s)
|
|
||||||
```
|
|
||||||
|
|
||||||
Output:
|
|
||||||
|
|
||||||
```
|
|
||||||
['a', 'b', 'c', 'd', 'e']
|
|
||||||
```
|
|
||||||
|
|
||||||
## 4. map()
|
|
||||||
|
|
||||||
This makes one-liners super easy in Python. `map()` takes a function in the first parameter and applies it to all values in the iterable in the second parameter.
|
|
||||||
|
|
||||||
```python
|
|
||||||
def readints(string):
|
|
||||||
print(list(map(int, string.split()))
|
|
||||||
readInts("1 2 3 4 5")
|
|
||||||
```
|
|
||||||
|
|
||||||
Output:
|
|
||||||
|
|
||||||
```
|
|
||||||
[1, 2, 3, 4, 5]
|
|
||||||
```
|
|
||||||
|
|
||||||
It's most useful in assigning variables easily when you know the format the input will be in.
|
|
||||||
|
|
||||||
```python
|
|
||||||
a, b = (map(int, input().split()))
|
|
||||||
```
|
|
||||||
|
|
||||||
## 5. List generators
|
|
||||||
|
|
||||||
You can generate a new list using inline `for`.
|
|
||||||
|
|
||||||
```python
|
|
||||||
array = [i for i in range(10)]
|
|
||||||
print(array)
|
|
||||||
```
|
|
||||||
|
|
||||||
Output:
|
|
||||||
|
|
||||||
```
|
|
||||||
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
|
|
||||||
```
|
|
||||||
|
|
||||||
## 6. One-line prefix sum array
|
|
||||||
|
|
||||||
A [prefix sum array](https://en.wikipedia.org/wiki/Prefix_sum) is a common data structure used in competitive programming. Python's `itertools` module has a wide array of functionality that can make this easier. A traditional PSA requires three lines:
|
|
||||||
|
|
||||||
```python
|
|
||||||
psa = [0]
|
|
||||||
for i in [1, 2, 3]:
|
|
||||||
psa.append(psa[-1] + i)
|
|
||||||
```
|
|
||||||
|
|
||||||
Excluding the import, you can shrink it down to one with `itertools`:
|
|
||||||
|
|
||||||
```python
|
|
||||||
import itertools
|
|
||||||
*psa, = itertools.accumulate([1, 2, 3])
|
|
||||||
```
|
|
||||||
|
|
@ -1,155 +0,0 @@
|
|||||||
---
|
|
||||||
title: Primoprod Progress Report
|
|
||||||
date: 2021-08-21
|
|
||||||
tags:
|
|
||||||
- primoprod
|
|
||||||
- tech
|
|
||||||
- blog
|
|
||||||
---
|
|
||||||
|
|
||||||
Welcome to the very first [Primoprod](https://primoprod.eggworld.tk) progress report! In a similar vein to quite a few open source emulation projects (such as those I follow myself using [emufeed](https://github.com/potatoeggy/emufeed/blob/master/sources.py)), I'll be releasing these tidbits in lieu of daily Unstagnation shorts sometimes.
|
|
||||||
|
|
||||||
In this hopefully small series of development notes, I'll be laying out my experiences learning web development as an absolute amateur.
|
|
||||||
|
|
||||||
This report will cover the beginnings of the project to the present day: 16 July - 20 August 2021.
|
|
||||||
|
|
||||||
<!-- excerpt -->
|
|
||||||
|
|
||||||
## Introduction
|
|
||||||
|
|
||||||
What is Primoprod? Short for "Productivity Primogems", it was born when I noticed that the gacha system employed by games such as *Genshin Impact* could be incredibly addictive, so I decided to see if I could take advantage of it to boost my productivity and at the same time try to learn web development.
|
|
||||||
|
|
||||||
The basic premise was to assign a given point value for each productive task and be able to spend those points on something or have them progress toward a milestone, so that productive tasks would be incentivised. The aforementioned gacha games and Uber use this to great effect, so I decided to emulate the Wish UI of [*Genshin Impact*](https://genshin.mihoyo.com/en).
|
|
||||||
|
|
||||||
And so the project began! I decided to work with Vue.js because of its gentler learning curve compared to Angular and more traditional HTML/CSS/JS separation compared to React. You can tell when I became more comfortable in using Vue's declarative system in the later components compared to, say, [`App.vue`](https://github.com/potatoeggy/primoprod/blob/master/src/App.vue).
|
|
||||||
|
|
||||||
Of course, the project was made open source on [GitHub](https://github.com/potatoeggy/primoprod) and licensed under the [GNU AGPLv3](https://en.wikipedia.org/wiki/GNU_Affero_General_Public_License) so that anyone can use it. (Except for the assets, but we'll get to that later.)
|
|
||||||
|
|
||||||
## First steps
|
|
||||||
|
|
||||||
As my first foray into web development, there were many tools and practices I could choose from. Vue's justification was outlined in the introduction, but I had no clue how Webpack worked, what folder structure I should use, how I should lay out my code, etc.
|
|
||||||
|
|
||||||
Luckily, I didn't have to make any of these decisions because Vue's [CLI](https://cli.vuejs.org/) gives you a list of sane defaults that you can pick from, and since I was learning for the future, I went with the first option of Vue 3 + Typescript. `vue-cli` even nicely [initialised a git repo for me](https://github.com/potatoeggy/primoprod/commit/9b7d7841806c905e8f580f98d1c95d4732178810)!
|
|
||||||
|
|
||||||
At the time, coming from Python/Java, I opted in to the class components plugin hoping it made it easier to develop for, but later removed it due to a lack of documentation with it for Vue 3. Occasional downsides of newer technologies ¯\\\_(ツ)_/¯.
|
|
||||||
|
|
||||||
The [first few commits](https://github.com/potatoeggy/primoprod/commit/ed9d94b61bf91ea9b82ac4d832dfb2b9ff2efc59) had me playing around until I was comfortable enough to introduce my very [first component](https://github.com/potatoeggy/primoprod/commit/fcbb4068dd3b018db2809ccfcc5381d4ea3ae727): the WishButton.
|
|
||||||
|
|
||||||
{% asset_img "wish-button-emulated.png" %}
|
|
||||||
|
|
||||||
I'd say it turned out pretty well! Since I wanted to emulate Genshin's UI, I wanted to match it as closely as I could. These two buttons are made of an image inside of a div relatively positioned with text absolutely positioned inside. Original image for comparison:
|
|
||||||
|
|
||||||
{% asset_img "wish-button-original.png" %}
|
|
||||||
|
|
||||||
There are still some differences between the texts since Genshin uses antialiasing, and the alignment and shadow of the icon beside the wish quantity is slightly off too, but I would consider this result to be acceptable.
|
|
||||||
|
|
||||||
## CSS pain
|
|
||||||
|
|
||||||
When working with "pure" Vue, the hard part is not usually HTML, which is quite simple and is usually obvious if something is wrong, nor is it Vue/TypeScript itself — I know TypeScript saved me some pain by doing type checking prior to runtime several times, even — but instead CSS.
|
|
||||||
|
|
||||||
CSS.
|
|
||||||
|
|
||||||
No, it's not that it was difficult to center or align a `div`, generally (slap everything in flexboxes and magic happens), but the time spent getting the wish buttons to look exactly how I wanted to would be nothing compared to some of the later parts.
|
|
||||||
|
|
||||||
No one warns you about this stuff either; all the memes are about JS not making sense but no one talks about CSS except being hard to center divs!
|
|
||||||
|
|
||||||
See [GemCounter](https://github.com/potatoeggy/primoprod/blob/master/src/components/GemCounter.vue), the second component I made. All those precise margins/padding…
|
|
||||||
|
|
||||||
Although I had read up on [MDN's](https://developer.mozilla.org/en-US/) fantastic tutorials/documentation a fair bit and used flexboxes and `rem` everywhere, I apparently did not catch `box-sizing: border-box` and the margins and padding just did not arrange themselves how they should have.
|
|
||||||
|
|
||||||
{% asset_img "mdn-box-sizing-tip.png" %}
|
|
||||||
|
|
||||||
:/ thanks MDN for letting me know
|
|
||||||
|
|
||||||
[Some foreshadowing](https://github.com/potatoeggy/primoprod/blob/master/src/components/ItemRevealScreen.vue#L224)
|
|
||||||
|
|
||||||
## Smooth beginnings
|
|
||||||
|
|
||||||
Designing the basic screen was pretty straightforward. For all its woes, pure CSS still works and is intuitive enough that my git history was only slightly too messy and I got my results.
|
|
||||||
|
|
||||||
{% asset_img "primoprod-wishbanners.png" %}
|
|
||||||
|
|
||||||
Pretty good, right? Now, the design still isn't adaptive enough *since things get cut off for who knows why I thought flexboxes were supposed to solve all this* but for the most part it looks good enough. It appears I'll have to make a lot of exceptions for mobile devices…
|
|
||||||
|
|
||||||
{% asset_img "primoprod-wishbanners-scaled.png" %}
|
|
||||||
|
|
||||||
With some help taken by examining the assets of https://genshin.thekima.com and https://gi-wish-simulator.uzairashraf.dev, I grabbed a static background image as well as the videos!
|
|
||||||
|
|
||||||
For now, every time you pressed the wish button, you got a five-star animation.
|
|
||||||
|
|
||||||
## Asset prefetching and inconsistencies
|
|
||||||
|
|
||||||
Progress was steady and things appeared to be running perfectly. but on my local machine things load instantly, courtesy of it not being beamed around the world. So when it was deployed to https://primoprod.eggworld.tk, even with a relatively fast download speed, the background image loaded slowly, and there were a few seconds of downtime before a video would play.
|
|
||||||
|
|
||||||
This was unacceptable, so I investigated around on how Vue prefetches assets before giving up and setting up an [Electron](https://www.electronjs.org/) build for the desktop — this doesn't resolve the issue on the web, but until I'm more competent with Vue I can't quite make the necessary changes. The issue of slow asset loading also severely lengthened the suffering in the `ItemRevealScreen.vue` arc, where animations and audio had to be timed…
|
|
||||||
|
|
||||||
## Gacha and storage backend
|
|
||||||
|
|
||||||
And with the basic frontend done, a backend would be needed to determine what items were pulled, and they had to be stored in a compact way.
|
|
||||||
|
|
||||||
I opted for a [standard item database](https://github.com/potatoeggy/primoprod/blob/master/src/banners/ItemDatabase.json) accessible from anywhere that would make it easy to add/remove/reference characters in the future. To conserve storage space on the user's end (why do I bother, really), items are stored as [string IDs](https://github.com/potatoeggy/primoprod/blob/master/src/banners/Inventory.ts) and the inventory class has a plethora of helper functions to work with.
|
|
||||||
|
|
||||||
The [gacha](https://github.com/potatoeggy/primoprod/blob/master/src/banners/Gacha.ts) file was heavily inspired (and many ideas taken from) https://github.com/uzair-ashraf/genshin-impact-wish-simulator/blob/master/src/models/base-gacha.js, many thanks! Because we don't need the previous-banner-switching capability that it uses, I stripped out the unnecessary bits and kept it simple-ish.
|
|
||||||
|
|
||||||
As Primoprod evolved and grew past my initial design of random bullshit go!, these critical classes went through some changes that added functions such as being able to calculate the extra stardust/starglitter rewards from a gacha. This let me keep banners to be comparatively simple, defined in [easy-to-read and copy JSON](https://github.com/potatoeggy/primoprod/blob/master/src/banners/tapestry-of-golden-flames.json).
|
|
||||||
|
|
||||||
Typescript was actually really helpful here, since as a nearly complete newcomer to JavaScript, it made sure I was doing legal things — something I also found a little annoying in Python.
|
|
||||||
|
|
||||||
Just my opinion, but I much prefer statically typed languages such as C#/Java — not Java screw Java.
|
|
||||||
|
|
||||||
## ItemRevealScreen horror
|
|
||||||
|
|
||||||
Everything had gone extremely smoothly up to this point. I was making rapid progress, even with the time spent on Stack Overflow to learn CSS and JavaScript.
|
|
||||||
|
|
||||||
But then came the pull screen.
|
|
||||||
|
|
||||||
https://youtu.be/g1jDTHCRyCY?t=165
|
|
||||||
|
|
||||||
It looks simple, right? Deceptively simple. But there's a lot going on here.
|
|
||||||
|
|
||||||
There is:
|
|
||||||
|
|
||||||
- a zoom-in of the character image, followed by
|
|
||||||
- a slide-right of the character image, while
|
|
||||||
- the text slides left, while-after
|
|
||||||
- the element icon slides left, after which
|
|
||||||
- the stars appear in sequence, during? which
|
|
||||||
- the extra reward screen slides left.
|
|
||||||
|
|
||||||
Not to mention all of the glow and particle effects. All of these had to be aligned with audio, which I couldn't find after listening for hours on end to many of the sound effects in the game files so gave up and recorded my own pulls.
|
|
||||||
|
|
||||||
I don't know how actual developers take care of this — potentially a library — but aside from the alignment of everything (which was its own nightmare to be adaptable across multiple display sizes and I only finished everything but the extra slide yesterday), I spent waaaaaaay too long making Vue work with an "animation index" that tracked whose turn it was to show animations.
|
|
||||||
|
|
||||||
The lack of proper asset prefetching was also an issue after deployment since the images took time to load and the animation had already started, so even more went into `animationIndex` to check that the audio and images are loaded before playing.
|
|
||||||
|
|
||||||
The code got [progressively worse](https://github.com/potatoeggy/primoprod/commits/master/src/components/ItemRevealScreen.vue) and is now the [most complex](https://github.com/potatoeggy/primoprod/blob/master/src/components/ItemRevealScreen.vue) thing that I never want to touch again by far, but there's still more to do ;-;. Not to mention that if I need to add more animation indexes (say, if I need them for glow effects) I am utterly screwed unless I add a second animation index for those effects…
|
|
||||||
|
|
||||||
There are still occasional desyncs and alignment isn't actually completely fixed and the extra rewards side is the simplest I can get away with and the weapons are missing backgrounds and shadows and the element icon doesn't glow and the weapon element doesn't have a correct gradient since unfortunately CSS doesn't support recolouring images with gradients.
|
|
||||||
|
|
||||||
But hey, it looks good enough, right?
|
|
||||||
|
|
||||||
No it doesn't it's not even close but I'm not coming back to that
|
|
||||||
|
|
||||||
## Sweet release
|
|
||||||
|
|
||||||
Now that `ItemRevealScreen` is *done and over with and will not need any changes*, before making myself work on the equally fun part of the project that is `ItemRevealAllOverlay`, I opted for a break in `ItemObtainScreen` and `ItemDescriptionOverlay` and a one-week hiatus.
|
|
||||||
|
|
||||||
And they were easy! Easy and fun. It was blissful to be working with structured HTML and CSS again, and the animation pains there were *nothing* compared to the trauma of `ItemRevealScreen`.
|
|
||||||
|
|
||||||
In fact, I consider those two to be 100% done unless I can find a way to apply a border that looks exactly like the original game's that isn't an image.
|
|
||||||
|
|
||||||
But it looks great!
|
|
||||||
|
|
||||||
{% asset_img "itemdescriptionoverlay.png" %}
|
|
||||||
|
|
||||||
{% asset_img "itemobtainoverlay.png" %}
|
|
||||||
|
|
||||||
## Wrapping up
|
|
||||||
|
|
||||||
As it turns out, I'm not actually near good enough nor is web development interesting enough to be able to outline issues like the emulator and Asahi Linux developers.
|
|
||||||
|
|
||||||
In fact, this was less a progress report and more complaining like a development log.
|
|
||||||
|
|
||||||
As such, every future version will be called a development log since I'm far too lazy to change the title now.
|
|
||||||
|
|
||||||
Until next time!
|
|
@ -1,31 +0,0 @@
|
|||||||
---
|
|
||||||
title: "Using IWD with YRDSB Wi-Fi"
|
|
||||||
date: "2021-09-14"
|
|
||||||
tags:
|
|
||||||
- blog
|
|
||||||
- tech
|
|
||||||
---
|
|
||||||
|
|
||||||
The iNet Wireless Daemon (iwd) is a lightweight and stable Wi-Fi manager for Linux systems. However, some configuration is needed for it to work properly on WPA Enterprise (802.1X) networks. For YRDSB:
|
|
||||||
|
|
||||||
<!-- excerpt -->
|
|
||||||
|
|
||||||
Create the file with the following contents at `/var/lib/iwd/<ssid>.8021x`
|
|
||||||
|
|
||||||
```ini
|
|
||||||
[Security]
|
|
||||||
EAP-Method=PEAP
|
|
||||||
EAP-Identity=<username>
|
|
||||||
EAP-PEAP-Phase2-Method=MSCHAPV2
|
|
||||||
EAP-PEAP-Phase2-Identity=<username>
|
|
||||||
EAP-PEAP-Phase2-Password=<password>
|
|
||||||
|
|
||||||
[Settings]
|
|
||||||
AutoConnect=true
|
|
||||||
```
|
|
||||||
|
|
||||||
…and then connect to the network normally.
|
|
||||||
|
|
||||||
```
|
|
||||||
iwctl station wlan0 connect YRDSB-S
|
|
||||||
```
|
|
@ -1,75 +0,0 @@
|
|||||||
---
|
|
||||||
title: "3 Reasons to Switch to Linux"
|
|
||||||
date: "2021-10-30"
|
|
||||||
tags:
|
|
||||||
- blog
|
|
||||||
- tech
|
|
||||||
---
|
|
||||||
|
|
||||||
In the computing world, Linux is already extremely widespread — it's near-ubiquitous on servers as well as many appliances such as smart fridges, [cars](https://www.zdnet.com/article/tesla-starts-to-release-its-cars-open-source-linux-software-code/), or even [Mars helicopters](https://www.theverge.com/2021/2/19/22291324/linux-perseverance-mars-curiosity-ingenuity) . If you have an Android phone, you're running Linux already. So why not give it a shot on your computer, too?
|
|
||||||
|
|
||||||
<!-- excerpt -->
|
|
||||||
|
|
||||||
First, what is Linux? At its heart, it is a [kernel](https://en.wikipedia.org/wiki/Kernel_(operating_system)) that the rest of your operating system and software depends on, from drivers to the display manager to games.
|
|
||||||
|
|
||||||
However, this article will largely focus on **desktop Linux**, which competes with other operating systems such as Windows and macOS.
|
|
||||||
|
|
||||||
# Complete freedom
|
|
||||||
|
|
||||||
Perhaps the biggest feature of Linux is its ability to do whatever you want, however you want. After a *tiny* bit of tinkering, you'll be able to set up your computer exactly how you'd like it!
|
|
||||||
|
|
||||||
{% asset_img "sway-desktop.png" %}
|
|
||||||
|
|
||||||
(caption: a terminal, an emulated Switch game, a game launcher, and a browser all automagically arranged by a tiling window manager. Click to enlarge. The currently playing song is in the top bar.)
|
|
||||||
|
|
||||||
Or, if you aren't the type to spend hours fiddling every little thing, you can choose from a variety of existing default desktop interfaces.
|
|
||||||
|
|
||||||
Try GNOME's minimalistic and beautiful desktop with its well-integrated virtual workspaces:
|
|
||||||
|
|
||||||

|
|
||||||
|
|
||||||

|
|
||||||
|
|
||||||
Or Plasma's endless customisation:
|
|
||||||
|
|
||||||

|
|
||||||
|
|
||||||

|
|
||||||
|
|
||||||
And this is only the beginning — it's not just appearance you have control over, although both GNOME and Plasma also come with their assortment of applications that have designs that perfectly mesh with the desktop, with global theming letting you click a single button in your settings menu to change colours or styles across all your apps.
|
|
||||||
|
|
||||||
Don't like your file manager? Swap it out for one of the dozens out there. Don't like how the update manager churns in the background? Turn it off! Or heck, even switch to another flavour of Linux that doesn't ask you to update so often. The sky's the limit!
|
|
||||||
|
|
||||||
**You can do anything.**
|
|
||||||
|
|
||||||
# The package manager
|
|
||||||
|
|
||||||
Speaking of the update manager…
|
|
||||||
|
|
||||||
Take a look at your phone and how you get new software or update old software — the App Store on iPhones and Google Play on Android phones. Now imagine being able to do that…on your computer. Being able to install whatever you want using a store with built-in security along with updates.
|
|
||||||
|
|
||||||
No more downloading random apps from the internet and hoping they aren't infected!
|
|
||||||
|
|
||||||
Not only that, but you can also update your computer *while* you're using it — no more waiting ages for Windows to finish doing what it does — most of the time you don't even need to reboot, but when you do it's just as fast as when you turn your computer on normally.
|
|
||||||
|
|
||||||
Here are just a couple of the graphical stores available:
|
|
||||||
|
|
||||||
GNOME Software for GNOME:
|
|
||||||
|
|
||||||
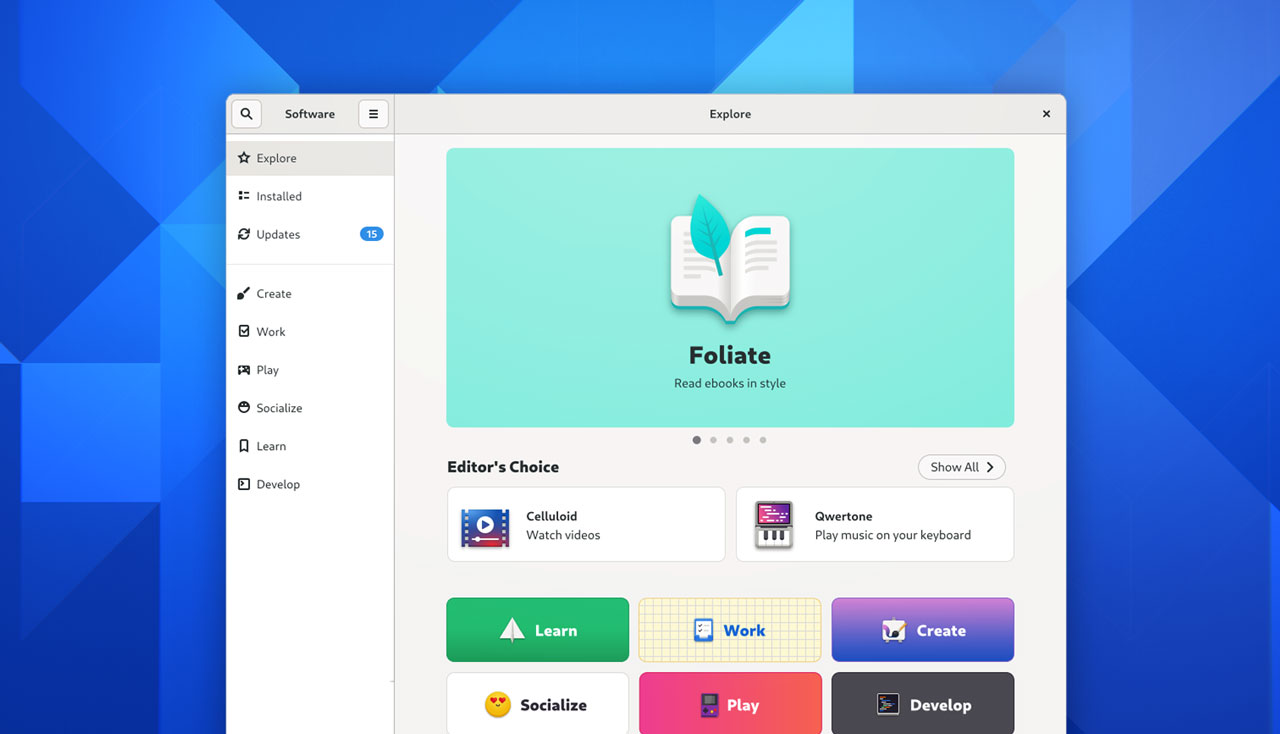
|
|
||||||
|
|
||||||
Discover for Plasma:
|
|
||||||
|
|
||||||
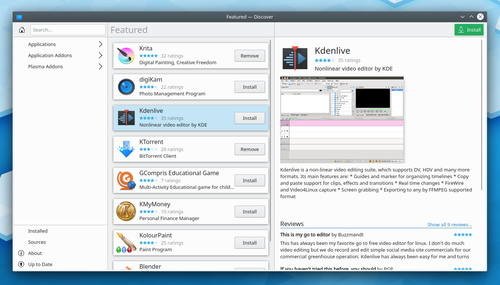
|
|
||||||
|
|
||||||
By contrast, the Microsoft Store was (is) a complete and utter mess that is nowhere near the integration and experience Linux has had for decades.
|
|
||||||
|
|
||||||
# Open source
|
|
||||||
|
|
||||||
Not only that, desktop Linux was built by thousands of volunteers, each contributing their own code to make the best product they can. Because it's completely open source (anyone can see or edit the source code), it's inherently more secure as simply more people are looking at it to fix issues and squash bugs.
|
|
||||||
|
|
||||||
Learning Linux is a great opportunity to jump into learning more about computers because of the knowledge you gain over time of how your computer works on a fundamental level as you inevitably start troubleshooting *when* something breaks. And perhaps you'll be the one to contribute back upstream to the project too, if you fix a bug or add a new feature, and have your own code distributed around to millions of other users.
|
|
||||||
|
|
||||||
# Try it now!
|
|
||||||
|
|
||||||
With dozens of well-maintained versions of Linux operating systems out there, you'll be sure to find one that suits your needs. To try GNOME, [Pop!_OS](https://pop.system76.com/) or [Fedora](https://getfedora.org/en/workstation/download/) provide a seamless out-of-the-box experience. To try Plasma, [Kubuntu](https://kubuntu.org/) is a fantastic starting point. To get a macOS-like feel, [Elementary OS](https://elementary.io/) gives you that Apple vibe while, like every other Linux OS, is completely free of charge, and lets you try it out before you decide to install it.
|
|
@ -1,151 +0,0 @@
|
|||||||
---
|
|
||||||
title: "Primoprod Progress Report 2"
|
|
||||||
date: "2022-01-15"
|
|
||||||
tags:
|
|
||||||
- blog
|
|
||||||
- tech
|
|
||||||
- primoprod
|
|
||||||
---
|
|
||||||
|
|
||||||
Six months have passed since the [first progress report](https://eggworld.tk/posts/2021/08/primoprod-progress-report/). Since then, a flood of changes have made it in, including hundredth-class Android support!
|
|
||||||
|
|
||||||
This report will cover from where the previous left off to the present day: 21 August 2021 - 15 January 2022.
|
|
||||||
|
|
||||||
<!-- excerpt -->
|
|
||||||
|
|
||||||
## No more
|
|
||||||
|
|
||||||
{% asset_img "primoprod-itemrevealscreen.png" %}
|
|
||||||
|
|
||||||
It's done. The pull screen is done. The element/weapon icon was added to the pull. Audio syncs up (well enough). The only thing missing is all of the fancy effects like glow and particles.
|
|
||||||
|
|
||||||
Nah, this is good enough.
|
|
||||||
|
|
||||||
## Take this!
|
|
||||||
|
|
||||||
{% asset_img "primoprod-questscreen.png" %}
|
|
||||||
|
|
||||||
Until now, you had to manually edit the browser's `localStorage` to gain any currency. The quest screen makes primoprod finally usable as now you can make your own long-term "quests" that give 900 Primogems each as well as set four daily "tasks" that give 30 Primogems each plus 60 when all are done — if only the base game was this generous. These are editable and can have whatever title or description you want. The logic here went through several rewrites as the structure was finalised and an interface developed to the rest of primoprod. Dailies will automatically refresh themselves on the next day.
|
|
||||||
|
|
||||||
In addition, there are several more features hidden in the Quest interface, such as an expiry time that you can't currently set.
|
|
||||||
|
|
||||||
By the way, JavaScript really doesn't have a good way to set an exact date relative to today — in the end I had to resort to *this* to set an expiry time for dailies.
|
|
||||||
|
|
||||||
```js
|
|
||||||
expires: (() => {
|
|
||||||
const d = new Date();
|
|
||||||
d.setDate(d.getDate() + 1);
|
|
||||||
d.setHours(4, 0, 0, 0);
|
|
||||||
return d;
|
|
||||||
// somehow this way is actually nicer than the new Date() way
|
|
||||||
})(),
|
|
||||||
```
|
|
||||||
|
|
||||||
## First pre-release
|
|
||||||
|
|
||||||
The [very first pre-release](https://github.com/potatoeggy/primoprod/releases/tag/1.0-beta1) of primoprod, **1.0-beta1**, was published on 25 August 2021.
|
|
||||||
|
|
||||||
It would be a few more months before the first stable release would be sent out into the world.
|
|
||||||
|
|
||||||
## Look to the future
|
|
||||||
|
|
||||||
Up to now, you might have noticed a considerable lack of pain in this progress report compared to the previous one. And for good reason: I got smarter.
|
|
||||||
|
|
||||||
Sounds incredible, right? As it turns out, as you gain more experience with technologies, you make less mistakes and follow best practices more.
|
|
||||||
|
|
||||||
This is why [the shop](https://github.com/potatoeggy/primoprod/blob/master/src/components/ShopScreen.vue) and the [dialog to buy things from the shop](https://github.com/potatoeggy/primoprod/blob/master/src/components/ItemPurchaseOverlay.vue) are so nicely done! It reused most of my types and was admittedly much simpler than some of the other screens, but I only ran into one insurmountable problem: range styling.
|
|
||||||
|
|
||||||
{% asset_img "primoprod-itempurchaseoverlay.png" %}
|
|
||||||
|
|
||||||
*I wish I was actually this rich in the base game.*
|
|
||||||
|
|
||||||
As you can see, the slider looks very out of place. Why? That's because [only Firefox](https://developer.mozilla.org/en-US/docs/Web/CSS/::-moz-range-progress) supports the needed CSS attribute to style the coloured bit before the "thumb" of the slider. I could make up something like Spotify and other services do using JavaScript, but that's a job for my future self.
|
|
||||||
|
|
||||||
## Pick your poison
|
|
||||||
|
|
||||||
Up until now, only one banner was supported. This was finally fixed in November with the addition of [banner headers](https://github.com/potatoeggy/primoprod/pull/25) to match the base game. Now, you can simultaneously roll for Qiqi on *both* banners!
|
|
||||||
|
|
||||||

|
|
||||||
|
|
||||||
On a side note, did you know that Vue puts all of their reactive things into Proxies? This means you can't simply `console.log(obj)` without going through five more clicks to find what you actually want. No, no. To properly print out the actual object, you have to *copy its contents to a clean, non-reactive Object* for this to work. Why??
|
|
||||||
|
|
||||||
```js
|
|
||||||
console.log("Rolled:", this.lastRoll); // nope
|
|
||||||
```
|
|
||||||
|
|
||||||
```js
|
|
||||||
console.log("Rolled:", [...this.lastRoll.map((i) => Object.assign({}, i))]); // thank you vue love that
|
|
||||||
```
|
|
||||||
|
|
||||||
## First release
|
|
||||||
|
|
||||||
After 8 beta pre-releases, [version 1.0.0](https://github.com/potatoeggy/primoprod/releases/tag/1.0.0) of Primoprod was successfully launched on 15 December 2021! I'm happy to tell you that the release went perfectly, was completely stable, and had absolutely no bugs whatsoever.
|
|
||||||
|
|
||||||
None.
|
|
||||||
|
|
||||||
None at all.
|
|
||||||
|
|
||||||
## Imagine not properly structuring your programs
|
|
||||||
|
|
||||||
Thanks to my astute design of primoprod, there were zero problems when the base game switched to having [double event banners](https://github.com/potatoeggy/primoprod/releases/tag/1.0-beta8) — they would simply share the same banner storage. Truly a stroke of genius. Practically a zero-line change.
|
|
||||||
|
|
||||||
The thing that *did* trip me up was the requirement for descriptions — this required all banners to be [updated](https://github.com/potatoeggy/primoprod/commit/12e7decdc6f5724afda467d6977d566b5c762e2e) to include a description as well as version upgrade code to migrate the pull data from older versions of primoprod into 1.0.1 without issues.
|
|
||||||
|
|
||||||
```js
|
|
||||||
switch (localStorage.version) {
|
|
||||||
case undefined: // pre-1.0.1: Add descriptions
|
|
||||||
if (localStorage.pullHistory) {
|
|
||||||
localStorage.pullHistory = JSON.stringify(
|
|
||||||
JSON.parse(localStorage.pullHistory).map((pull: Pull) => {
|
|
||||||
pull.description =
|
|
||||||
pull.bannerStorage === "event"
|
|
||||||
? "Character Event Wish"
|
|
||||||
: "Permanent Wish";
|
|
||||||
return pull;
|
|
||||||
})
|
|
||||||
);
|
|
||||||
}
|
|
||||||
console.log("Updated from pre-1.0.1 to 1.0.1");
|
|
||||||
}
|
|
||||||
localStorage.version = 1;
|
|
||||||
```
|
|
||||||
|
|
||||||
In hindsight, simply linking the banner they came from to this would have saved more data as well as have been more future-proof.
|
|
||||||
|
|
||||||
## Can't stop, won't stop
|
|
||||||
|
|
||||||
By this point, I'd become a little fed up with having to wait several minutes for my computer to build primoprod each release every two weeks, so I looked into using GitHub Actions for continuous integration.
|
|
||||||
|
|
||||||
Several days of tweaking later, I got GitHub to successfully [build and upload](https://github.com/potatoeggy/primoprod/blob/master/.github/workflows/build.yml) artifacts on each commit for all three operating systems — but they were zipped (even if they were just one file) and couldn't be attached to a release…
|
|
||||||
|
|
||||||
Back to laptop compilation we go!
|
|
||||||
|
|
||||||
## Pretty badges
|
|
||||||
|
|
||||||
Obviously, you aren't a *real* free and open source project if you don't have [pretty badges](https://github.com/potatoeggy/primoprod/blob/master/README.md) on your README. This was a major concern, so I copied Vue's style and now made primoprod a proper FOSS repo — build checkmark and badges and all!
|
|
||||||
|
|
||||||
{% asset_img "primoprod-badges.png" %}
|
|
||||||
|
|
||||||
## Kids and their phones
|
|
||||||
|
|
||||||
Annoying as it might be, many people only have phones and browse most often on their phones, so this project has to be mobile compatible. If you saw the last progress report, you know that mobile support was…lacking.
|
|
||||||
|
|
||||||
To remedy that for the 1.1.0 release, I spent a few days grinding out and cursing CSS as I was forced to go back to
|
|
||||||
|
|
||||||
ItemRevealScreen? What's that? Now [WishBanners](https://github.com/potatoeggy/primoprod/blob/v1.1.0/src/components/WishBanners.vue) is my enemy.
|
|
||||||
|
|
||||||
At last, though, we have a [proper mobile UI](https://github.com/potatoeggy/primoprod/pull/33).
|
|
||||||
|
|
||||||
{% asset_img "mobile-primoprod.png" %}
|
|
||||||
|
|
||||||
Still some niggles to work out, but it looks "good enough"! With the completion of proper mobile orientation came the merging of the [Android branch](https://github.com/potatoeggy/primoprod/pull/32) made with [Capacitor.js](https://capacitorjs.com/), basically the mobile equivalent of Electron. It has even more niggles than the web version does.
|
|
||||||
|
|
||||||
If you have any idea how to fix them, please do send a suggestion on the [issue tracker!](https://github.com/potatoeggy/primoprod/issues/34)
|
|
||||||
|
|
||||||
## Present day
|
|
||||||
|
|
||||||
And that's everything that changed since August! Primoprod has come a long way from being a mere personal project to a proper personal project with fancy badges! Hopefully in the future it'll be able to be played standalone (chibi sprite combat, anyone?) so that it'll be interesting enough that people can set their goals and stick to them.
|
|
||||||
|
|
||||||
In case you'd like to check it out, version 1.1.0 released just [yesterday](https://github.com/potatoeggy/primoprod/releases/tag/v1.1.0) and is available for download for Windows, macOS, Linux, and Android, with a web version available at https://primoprod.eggworld.tk!
|
|
||||||
|
|
||||||
Until next time!
|
|
@ -1,269 +0,0 @@
|
|||||||
---
|
|
||||||
title: "BSSCC Linux Scavenger Hunt - Solutions"
|
|
||||||
date: "2022-04-07"
|
|
||||||
tags:
|
|
||||||
- blog
|
|
||||||
- tech
|
|
||||||
- bsscc
|
|
||||||
---
|
|
||||||
|
|
||||||
SPOILERS if you haven't completed it.
|
|
||||||
|
|
||||||
<!-- excerpt -->
|
|
||||||
|
|
||||||
Assume all part 1 commands here are run in the home directory. Assume all part 2 commands are run in the `part2` directory.
|
|
||||||
|
|
||||||
## Clue 1
|
|
||||||
|
|
||||||
#### Solution
|
|
||||||
|
|
||||||
```bash
|
|
||||||
ls
|
|
||||||
```
|
|
||||||
|
|
||||||
No explanation necessary here. Clue 1 is in the file name itself, so simplying listing the files in the home directory will return the first clue.
|
|
||||||
|
|
||||||
## Clue 2
|
|
||||||
|
|
||||||
#### Solution
|
|
||||||
|
|
||||||
```bash
|
|
||||||
cat clue2.txt
|
|
||||||
```
|
|
||||||
|
|
||||||
Presumably, after you `ls` and find the first clue, you would notice a file named `clue2.txt`, in which the second clue is contained.
|
|
||||||
|
|
||||||
## Clue 3
|
|
||||||
|
|
||||||
#### Solution
|
|
||||||
|
|
||||||
```bash
|
|
||||||
cd clue3
|
|
||||||
ls
|
|
||||||
cd "down here"
|
|
||||||
ls
|
|
||||||
cd "over here"
|
|
||||||
ls
|
|
||||||
cd "nearly there"
|
|
||||||
ls
|
|
||||||
cat "you found me!"
|
|
||||||
```
|
|
||||||
|
|
||||||
There are multiple ways to solve this problem, but the solution provided is the one that requires the least amount of knowledge. Helpful folder names should guide you to the final file containing the clue.
|
|
||||||
|
|
||||||
## Clue 4
|
|
||||||
|
|
||||||
#### Solution
|
|
||||||
|
|
||||||
```bash
|
|
||||||
ls -a
|
|
||||||
cat .clue4
|
|
||||||
```
|
|
||||||
|
|
||||||
The assist text at the end of clue 3 implies that you should return to your home directory, and that there are hidden files:
|
|
||||||
|
|
||||||
> The fourth clue is back at home, but you might need to look more closely for something that lies unseen...
|
|
||||||
|
|
||||||
…upon which you would discover three new files, each containing clues. Clue 4's only challenge is finding it.
|
|
||||||
|
|
||||||
## Clue 5
|
|
||||||
|
|
||||||
#### Solution
|
|
||||||
|
|
||||||
```bash
|
|
||||||
chmod +r .clue5
|
|
||||||
cat .clue5
|
|
||||||
```
|
|
||||||
|
|
||||||
Off the heels of clue 4, you would probably do the same thing. Unfortunately, there is something else you have to do:
|
|
||||||
|
|
||||||
```
|
|
||||||
cat: .clue5: Permission denied
|
|
||||||
```
|
|
||||||
|
|
||||||
This should be a hint that you have to muck around with permissions. As you're trying to read the file, you would add the *read* permission to it, which should then reveal the clue.
|
|
||||||
|
|
||||||
## Clue 6
|
|
||||||
|
|
||||||
#### Solution
|
|
||||||
|
|
||||||
```bash
|
|
||||||
grep NEEDLE .the_sixth_clue
|
|
||||||
```
|
|
||||||
|
|
||||||
Completing clue 5 is almost required to complete this clue with the following help text.
|
|
||||||
|
|
||||||
> Clue 6 is pretty big and hard to look through. Can you find the NEEDLE in the haystack?
|
|
||||||
|
|
||||||
There is no chance of you manually scrolling unless you want to scroll for a very long time — there are 10000 lines to scroll through, and the clue is found on line 777. Following the help text, *literally* finding the word "NEEDLE" will take you to the clue.
|
|
||||||
|
|
||||||
However, you might have noticed a pattern so far — each clue begins with "Clue X: cluehere", so you might be inclined to simply grep for the word "Clue". This does work for this clue and was used by many beta testers, but the shortcut means that you may struggle a little in the second half, which builds on this concept.
|
|
||||||
|
|
||||||
## Clue 7
|
|
||||||
|
|
||||||
#### Solution
|
|
||||||
|
|
||||||
```bash
|
|
||||||
chmod +x clue7
|
|
||||||
./clue7
|
|
||||||
```
|
|
||||||
|
|
||||||
Similar to clue 5, although you might try to read it, garbled text and the hint text from the clue unlocker used to unlock this clue should lead you to conclude that you should be running this program instead.
|
|
||||||
|
|
||||||
> About Clue 7: Fun fact: you can run anything on Linux. No need for a “.exe” or “.app” file extension. But what do you have to do to be able to run it…?
|
|
||||||
|
|
||||||
Therefore, once you get another permission denied trying to run it, you conclude that you have to once again set the executable bit with `chmod` and run it as a compiled C program.
|
|
||||||
|
|
||||||
## Clue 8
|
|
||||||
|
|
||||||
#### Solution
|
|
||||||
|
|
||||||
```bash
|
|
||||||
cd /
|
|
||||||
ls
|
|
||||||
cat clue8
|
|
||||||
```
|
|
||||||
|
|
||||||
> To find the eighth clue, you'll have to go up. All the way up.
|
|
||||||
|
|
||||||
"Going up" here can mean a variety of things, but at the knowledge level of the scavenger hunt should imply that you go up the *directory tree*, going "all the way up" to the root directory `/`, where looking around should reveal the `clue8` file containing the eighth clue.
|
|
||||||
|
|
||||||
## Clue 9
|
|
||||||
|
|
||||||
#### Solution
|
|
||||||
|
|
||||||
```bash
|
|
||||||
grep -vi needle clue9.txt
|
|
||||||
```
|
|
||||||
|
|
||||||
> About Clue 9: What if you’re looking for a clue in a haystack of needles? You'd have to take it all apart. You wouldn’t want to find a needle, after all. Regardless if the nEeDlES are big or if the neeDLES are small, you don't want any of them.
|
|
||||||
|
|
||||||
The puzzle here expands on that from the sixth clue and practically inverts it. Once again, you're searching for a clue, but instead of finding a clue using the needle, you want to find the line *without* the needle.
|
|
||||||
|
|
||||||
Trying to `grep` for "Clue" or ":" if you tried that in clue 6 will show you a line that kindly informs you that you should try a different approach.
|
|
||||||
|
|
||||||
```
|
|
||||||
Clue 9: Not this line ;) — try again! needle
|
|
||||||
```
|
|
||||||
|
|
||||||
Instead, the solution is to find every line that *does not* contain a needle case-insensitively.
|
|
||||||
|
|
||||||
```
|
|
||||||
Hint IX is as follows - cxS1YxZFl
|
|
||||||
```
|
|
||||||
|
|
||||||
## Clue 10
|
|
||||||
|
|
||||||
#### Solution
|
|
||||||
|
|
||||||
```bash
|
|
||||||
ls -R clue10
|
|
||||||
cat clue10/2/1/1/*
|
|
||||||
```
|
|
||||||
|
|
||||||
Much like how clue 9 expanded on clue 6, clue 10 expands on clue 3. This time, there are no hints and the number of folders is too large to reasonably manually `cd` and `ls` through, so you'll need to find another way around.
|
|
||||||
|
|
||||||
```bash
|
|
||||||
ls clue10
|
|
||||||
0 1 2 "don't bother trying to brute force this" 'you'\''ll have to put the pieces together - wild, huh?'
|
|
||||||
```
|
|
||||||
|
|
||||||
Practical solutions here involve `ls -R` but also `find .`, both of which will show all subdirectories in `clue10`, revealing 10 text files in `clue10/2/1/1` that must be combined to form the clue. The help folder in `clue10` hints that **wildcards** can be used to quickly see the contents of each file.
|
|
||||||
|
|
||||||
## Clue 11
|
|
||||||
|
|
||||||
#### Solution
|
|
||||||
|
|
||||||
```bash
|
|
||||||
unzip clue11.zip
|
|
||||||
cat clue11.txt
|
|
||||||
```
|
|
||||||
|
|
||||||
File extensions aren't completely useless — they can hint to what you might need to do if used properly. No explanation needed here.
|
|
||||||
|
|
||||||
## Clue 12
|
|
||||||
|
|
||||||
#### Solution
|
|
||||||
|
|
||||||
```bash
|
|
||||||
mv clue9.txt clue99.txt
|
|
||||||
cp ../clue2.txt clue100.txt
|
|
||||||
rm ../clueunlocker.pyc
|
|
||||||
mkdir bucket
|
|
||||||
python clue12.pyc
|
|
||||||
```
|
|
||||||
|
|
||||||
The last clue is the most complex clue. You will have to perform four file manipulations to get it, with plenty of messages if you do it wrong.
|
|
||||||
|
|
||||||
> Where's clue 99? Oh, it's called clue9 now... Would you be a dear and move it to clue99.txt?
|
|
||||||
|
|
||||||
The first task asks you to move the 9th clue to the 99th clue. Be sure not to copy it, or the program will get mad at you.
|
|
||||||
|
|
||||||
> Wait, there are supposed to be 100 clues!
|
|
||||||
> Just copy that second clue you got and put it here, alright?
|
|
||||||
> Name it clue100.txt and we'll call it even.
|
|
||||||
|
|
||||||
Clue 12 has developed an attitude and now wants you to bring `clue2.txt` to `clue100.txt`. Make sure to copy it *to the same directory as clue12*.
|
|
||||||
|
|
||||||
For fun, if you moved it instead of copying it:
|
|
||||||
|
|
||||||
> I told you to *copy* it, not move it! Do as I say next time or else I'm locking you out.
|
|
||||||
|
|
||||||
Or if you copied a different file / created a file named clue100.txt without copying clue2:
|
|
||||||
|
|
||||||
> You trying to scam me over here? I thought I told you to copy clue2.txt, not whatever garbage you dumped.
|
|
||||||
|
|
||||||
The third task simply wants you to remove `clueunlocker.pyc` in the home directory.
|
|
||||||
|
|
||||||
> Oi! What's that troublemaking clue unlocker doin' here? Get rid of 'im. Right now. I see 'im smirking in his little hidey-hole in your home. Delete him off of the face of the planet or I ain't giving you my clue.
|
|
||||||
|
|
||||||
Lastly, the (most difficult?) clue wants you to give it a bucket.
|
|
||||||
|
|
||||||
> At least that b****** clueunlocker.pyc ain't 'ere. I'd be doing bad things if 'e was here, I would.
|
|
||||||
> *cough* I ain't feelin' too good. Quick, fetch me a bucket, will ya? Put it right beside me when you're done. Make sure I know it's a bucket, just a bucket, my eyes do be swimmin' these days.
|
|
||||||
|
|
||||||
It wants a folder named `bucket` to vomit whatever it's having trouble with. If you happen to place a file named `bucket` instead, you'll be kindly informed that you might want to consider a folder instead.
|
|
||||||
|
|
||||||
> Wha... what is this? This ain't no bucket! Quick, before I -- BLERGH!
|
|
||||||
> .
|
|
||||||
> .
|
|
||||||
> You sense that your clue-holder won't be coming back anytime soon. Maybe you should consider giving him a more empty container.
|
|
||||||
|
|
||||||
With that, you finally receive the final clue!
|
|
||||||
|
|
||||||
> Whew, something came up. Bless /dev/null that there was this bucket here.
|
|
||||||
> Anyway, here you are. Don't let me hold you back, now.
|
|
||||||
> Submit your combined code to judge.bayview.club!
|
|
||||||
|
|
||||||
To wrap up the main hunt, you have to run your combined code through the base64 decoder outlined in the initial instructions:
|
|
||||||
|
|
||||||
```bash
|
|
||||||
echo CODE | base64 -d
|
|
||||||
```
|
|
||||||
|
|
||||||
This will earn you your prize, which you can claim at https://prize.bayview.club !
|
|
||||||
|
|
||||||
## Secret
|
|
||||||
|
|
||||||
#### Solution
|
|
||||||
|
|
||||||
```bash
|
|
||||||
echo <CODE> | base64 -d
|
|
||||||
echo <NEWCODE> | base64 -d
|
|
||||||
echo <NEWNEWCODE> | base64 -d
|
|
||||||
echo <NEWNEWNEWCODE> | base64 -d
|
|
||||||
echo <NEWNEWNEWNEWCODE> | base64 -d
|
|
||||||
```
|
|
||||||
There is one secret solution implied in the instructions:
|
|
||||||
|
|
||||||
> If you are so inclined, you may find that the base case is not the base it appears to be, and that there might be something deeper...
|
|
||||||
|
|
||||||
A "base case" is a term for when recursion ends and things start returning up the stack. The hint here implies that the base64 command you're given is *not* the end, and perhaps you can run it a few more times to get a different result.
|
|
||||||
|
|
||||||
To be precise, running the command five times on each code you get will give you:
|
|
||||||
|
|
||||||
```
|
|
||||||
you found a secret!
|
|
||||||
```
|
|
||||||
|
|
||||||
Submitting this to the judge will grant you a score of 3/3 and access to the secret prize: https://prize.bayview.club/secret .
|
|
@ -1,9 +0,0 @@
|
|||||||
---
|
|
||||||
title: Run On
|
|
||||||
date: 2020-04-16
|
|
||||||
tags:
|
|
||||||
- literature
|
|
||||||
---
|
|
||||||
|
|
||||||
I was running from a dark thing and it was all horrible when my eyes snapped open and I looked at my alarm clock, which said that it was ten in the morning, which meant that I was going to be late for school unless I teleported back in time, so I got up and rushed to put on my clothes and do everything, then I didn’t even eat breakfast as I rushed to school before realising I could use my magical powers that I could as soon as I turned eleven to turn back time and avoid all this mess, so that’s what I did, but only after I actually ate breakfast and took my time, then I looked deep into myself and used my powers from friendship and determination to go back three hours (my friends were the bestest ever after I saved their lives using my magical powers), and I walked calmly to school, trying not to blush at my boyfriend (who is actually the hottest werewolf ever) when he suddenly popped out of nowhere, and I clutched his hand because I loved him so much, then he said, “oh hi babe what’s up?” then I said, “oh nothing,” then I giggled because he was so funny and then he laughed because I laughed then I laughed because he was laughing because I was giggling and everything was awesome, until suddenly a tree burst from the ground and we couldn’t get to school because suddenly there were tree monsters everywhere and I got scared but I knew that John (the werewolf) would protect me because he was a werewolf and so he transformed into a blue wolf (the rarest and most powerful kind) and after that he used his magical powers to vaporise the tree monsters with flashes of light and they all disappeared, and then I hugged him because I was scared and I said, “I was scared, thank you,” to which he said, “oh it’s nothing I would always protect you, my love,” and then I giggled again because he was being so cute, so I stared into his brilliant blue eyes which I only just realised matched the colour of his wolf form and admired all his really big muscles which would save me from any tree monster, but then suddenly he tensed and I realised that he was tense so I ran behind him again and peeked out from behind his shoulder and saw that something terrible appeared (even worse than tree monsters!), it was a ghost, and that was the first time I saw my boyfriend scared, which I could feel using my psychic powers so I told him telepathically that it was going to be ok and I could handle it using my dark powers which were super effective against psychics, and I focused really hard but nothing happened, so I thought of the strong bond that I had with all my friends and begged them for their help because there was this ghost and it could destroy the world, then they sent all their power and my eyes glowed as I tapped into a part of my power that i had never seen before, but it was now unlocked because of my friends’ determination and courage that flowed through my veins, as a white light burst from my hands that I held outstretched in front of me when I realised that this was the dark thing I was running from in my dream this morning, which I would finally kill because of the power of my friends that I had to see at school today, and I shouted as I killed the ghost, “omae wa mo shindeiru,” and it exploded and so we celebrated with my friends and they were all super impressed as I told the story and smiled at my hot boyfriend.
|
|
||||||
|
|
@ -1,69 +0,0 @@
|
|||||||
---
|
|
||||||
title: A Snowball Grows
|
|
||||||
date: 2021-01-29
|
|
||||||
tags:
|
|
||||||
- poetry
|
|
||||||
- literature
|
|
||||||
---
|
|
||||||
|
|
||||||
White falls from the sky \
|
|
||||||
onto a mountain \
|
|
||||||
and so a snowball grows.
|
|
||||||
<!-- excerpt -->
|
|
||||||
|
|
||||||
White falls from the sky \
|
|
||||||
on the village beside \
|
|
||||||
but the snow is shoveled aside. \
|
|
||||||
People laugh and they play, \
|
|
||||||
pushing problems away \
|
|
||||||
as the snowball grows.
|
|
||||||
|
|
||||||
The white comes down heavier \
|
|
||||||
and the villagers cheer \
|
|
||||||
but the mayor sees the danger. \
|
|
||||||
He travels around \
|
|
||||||
telling the town \
|
|
||||||
as the snowball grows.
|
|
||||||
|
|
||||||
"It's just a snowball," one person says \
|
|
||||||
as they stare at their screen. \
|
|
||||||
"We'll take care of it later," another suggests, \
|
|
||||||
the paperwork beside them untouched. \
|
|
||||||
"It's not going to hit us," asserts a third \
|
|
||||||
as they walk into their door. \
|
|
||||||
"That's a fake snowball," somebody claims \
|
|
||||||
as they wear their red cap. \
|
|
||||||
"That's too much work," the last person scoffs \
|
|
||||||
in his house barren of items.
|
|
||||||
|
|
||||||
And so the snowball grows.
|
|
||||||
|
|
||||||
One day the sun rises \
|
|
||||||
but the snowball \
|
|
||||||
blocks its light \
|
|
||||||
and its shadow \
|
|
||||||
looms over \
|
|
||||||
the village.
|
|
||||||
|
|
||||||
Panic erupts — \
|
|
||||||
the villagers scramble \
|
|
||||||
up to the mountain \
|
|
||||||
to hack away at the snowball.
|
|
||||||
|
|
||||||
The white comes down faster — \
|
|
||||||
the villagers pray \
|
|
||||||
but the snowball \
|
|
||||||
continues to grow.
|
|
||||||
|
|
||||||
Finally — \
|
|
||||||
the snowball is freed.
|
|
||||||
|
|
||||||
One house is crushed. \
|
|
||||||
A sigh of relief, \
|
|
||||||
and everyone return \
|
|
||||||
to help rebuild the house.
|
|
||||||
|
|
||||||
The next year: \
|
|
||||||
white falls from the sky \
|
|
||||||
onto a mountain \
|
|
||||||
and so a snowball grows.
|
|
@ -1,5 +0,0 @@
|
|||||||
{
|
|
||||||
"layout": "post.liquid",
|
|
||||||
"tags": ["posts"],
|
|
||||||
"published": true
|
|
||||||
}
|
|
@ -1,49 +0,0 @@
|
|||||||
---
|
|
||||||
title: A Favour II
|
|
||||||
date: 2021-07-27
|
|
||||||
tags:
|
|
||||||
- unstagnation
|
|
||||||
- literature
|
|
||||||
---
|
|
||||||
|
|
||||||
*Ring…ring…ring…*
|
|
||||||
|
|
||||||
Yellow! Are you there?
|
|
||||||
|
|
||||||
<!-- excerpt -->
|
|
||||||
|
|
||||||
Sorry, it's not about League today, haha. I was just talking to Blue the other day.
|
|
||||||
|
|
||||||
Listen, I think something's up with him. His voice was weird and raspy and there was clearly glass breaking in the background. He said it was Fortnite? I remember you were his best friend in university; do you know why he'd suddenly need a lot of money, by any chance? I know he wouldn't do anything like…*that*, but…
|
|
||||||
|
|
||||||
Oh! That's good to hear. When my boyfriend dropped off the money he wanted, he mentioned that the place felt super shady, so I thought maybe…
|
|
||||||
|
|
||||||
I'm just worried about him, that's all.
|
|
||||||
|
|
||||||
Ha — you too? Of course, I'd do anything for you guys. What is it?
|
|
||||||
|
|
||||||
No, I can't play League today, I've told you I have a job! We already play with Green and the others every Saturday anyway…
|
|
||||||
|
|
||||||
Huh. That's odd. Uh…Blue actually asked for that much yesterday.
|
|
||||||
|
|
||||||
Ah, that makes sense! Y'know, for a moment there I almost thought… Sorry for doubting you, yeah of course I'll help! Homes are getting really expensive, I've heard.
|
|
||||||
|
|
||||||
Congratulations, by the way! What's the address of your new house? Wanna invite Blue and Green and the others for a housewarming party when you move in?
|
|
||||||
|
|
||||||
So true! When I moved into my first house, it was like a dream come true. So many rooms. And the kitchen… it was ten times better than the dingy one at our dorm… it has an island in the middle!
|
|
||||||
|
|
||||||
…An *island in the middle*!
|
|
||||||
|
|
||||||
Right, right, sorry. Yes. Direct deposit, I take it?
|
|
||||||
|
|
||||||
Jesus, do you still have your flip phone too? And a laptop as thick as our textbooks? It's time to join the civilised world, man! Get with the times! Even Purple is less boomer than you, and she was ten times the nerd you were in uni.
|
|
||||||
|
|
||||||
Yadda yadda yadda, direct deposit is totally safe now, no one's going to be spying on you or anything…except for the banks, heh.
|
|
||||||
|
|
||||||
All right, fine. Old-fashioned cash it is. It'll take me some time to clear it with my bank, so I think I can get it to you…on Friday? And you still live with your parents?
|
|
||||||
|
|
||||||
Gotcha. Dude, you're way too addicted to League.
|
|
||||||
|
|
||||||
Shit. Sorry, my boss is calling me. Should I call you back?
|
|
||||||
|
|
||||||
See ya!
|
|
@ -1,43 +0,0 @@
|
|||||||
---
|
|
||||||
title: A Favour
|
|
||||||
date: 2021-06-20
|
|
||||||
tags:
|
|
||||||
- unstagnation
|
|
||||||
- literature
|
|
||||||
---
|
|
||||||
|
|
||||||
*Ring…ring…ring…*
|
|
||||||
|
|
||||||
Hello?
|
|
||||||
|
|
||||||
<!-- excerpt -->
|
|
||||||
|
|
||||||
Oh, Blue! I almost didn't recognise your voice; it's been forever since we chatted! How've you been doing?
|
|
||||||
|
|
||||||
Yeah, I know you have work and all, but…it's been, what, a year since we last played League together and it'd be great to get together again — remember that time when you were killed by a minion at bottom —
|
|
||||||
|
|
||||||
You don't play anymore? That's…a shame. Right, work and everything. So, uh, what'd you want to talk about?
|
|
||||||
|
|
||||||
A favour? Sure! What is it?
|
|
||||||
|
|
||||||
Uh…I think that's fine, but how much?
|
|
||||||
|
|
||||||
*A hundred thousand* — damn, did you buy a house or something?
|
|
||||||
|
|
||||||
Come to think of it, your voice is kinda raspy, and you're coughing a lot too. Are you all right? Should I come over?
|
|
||||||
|
|
||||||
A small fever, eh? Oh well. If you insist... I'll send the money over to your bank account, don't spend it on drugs, hehe.
|
|
||||||
|
|
||||||
Hm? Why not? Direct deposit's really safe now, you know. Why would you want it —
|
|
||||||
|
|
||||||
Right. Sorry. Sure, I'll come by and drop it outside your door, your address is still the same, right?
|
|
||||||
|
|
||||||
Ah, let's see... 135 Redwood Drive, is that right? You know, are you sure you're all right? I think I hear something smashing in the background...
|
|
||||||
|
|
||||||
Oh, yeah. Ugh, Fortnite. Right, I'll get the cash ready... I still don't know about dropping it off in an envelope; what if someone steals it? When would be a good time for you to pick it up? I'll swing by around that time if I can make it...
|
|
||||||
|
|
||||||
As soon as possible? Of course, Blue, my boyfriend's going to do groceries today; I'll send him your way at around...hm, three o'clock? Sounds good?
|
|
||||||
|
|
||||||
No problem! Friends gotta help each other out, right? I still wish we could play some League together, though...
|
|
||||||
|
|
||||||
Yeah, yeah, lots of work and everything. Well, you take care, Blue!
|
|
@ -1,26 +0,0 @@
|
|||||||
---
|
|
||||||
title: A Letter from Weilam to Ciers
|
|
||||||
date: 2020-08-20
|
|
||||||
tags:
|
|
||||||
- barin
|
|
||||||
- unstagnation
|
|
||||||
- literature
|
|
||||||
---
|
|
||||||
Saiyu Sphere\
|
|
||||||
1 Indigo Boulevard\
|
|
||||||
Saiyu, Weilam
|
|
||||||
<!-- excerpt -->
|
|
||||||
|
|
||||||
Mrs. Rio Nohigi\
|
|
||||||
Prime Minister\
|
|
||||||
1 Apelio Avenue\
|
|
||||||
Ciers
|
|
||||||
|
|
||||||
This letter is to inform you that Eos will not be able to hold this year's Continental Summit. The explosion detonated by Ptuyo has crippled large parts of the tribe, and I am deeply sorry to determine that this makes Eos unsafe for a gathering of world leaders. As per section 6 of the Saiyu Peace Agreement, responsibility for the organisation of the Summit will be transferred to Ciers.
|
|
||||||
|
|
||||||
This letter was sent on behalf of Eos by accelerated approval from the Ean High Representative of the Enigmatic Council.
|
|
||||||
|
|
||||||
Best regards,\
|
|
||||||
Brendan May\
|
|
||||||
Chairman of the Enigma Alliance\
|
|
||||||
20 August 2020
|
|
@ -1,36 +0,0 @@
|
|||||||
---
|
|
||||||
title: A Normal Morning in Xunil
|
|
||||||
date: 2020-06-20
|
|
||||||
tags:
|
|
||||||
- barin
|
|
||||||
- unstagnation
|
|
||||||
- literature
|
|
||||||
---
|
|
||||||
|
|
||||||
Light from the morning sun gently shone across my face as my curtains automatically opened to the start of the day. A steady beeping infiltrated my ears, rousing me from my sleep.
|
|
||||||
<!-- excerpt -->
|
|
||||||
|
|
||||||
“Good morning, Alston. It is seven in the morning.”
|
|
||||||
|
|
||||||
I rubbed my eyes and sat up, stretching to get the sleep out of my muscles. “Good morning to you too, Bixby. What do I have on my schedule today?”
|
|
||||||
|
|
||||||
As I headed to my bathroom to freshen up leaving my house, my personal assistant said, “You have: one meeting with the Technological Officer of Demauge to discuss continuing exchanges of technology and information between Demauge and Xunil.”
|
|
||||||
|
|
||||||
I looked up from the heated toilet seat I had just sat down on. “Is that it?”
|
|
||||||
|
|
||||||
“Yes.”
|
|
||||||
|
|
||||||
“So an easy day, then.” I finished defecating into the fancy lavatory and grabbed my electric toothbrush. The aforementioned fancy lavatory closed and cleaned itself as I left it. “Itsh been a while shinch I got won.”
|
|
||||||
|
|
||||||
Finishing my preparations for the day, I walked through the automatic door and to the people mover. As the machine carried me down to the lobby, I checked the status of and registered a route to the nearest train station.
|
|
||||||
|
|
||||||
The people mover dropped me off in front of the bus station outside. Just in time, too — I could see it arriving on the tracks. Putting my phone away, I speedwalked towards the escalator and took the steps two at a time. My digital identification opened the security barrier in advance, allowing me to catch the bus within my allotted boarding time.
|
|
||||||
|
|
||||||
When I reached the subway station, there was a surprising number of people crowding the escalators — some appeared to be holding signs, a protest maybe? — so I hurried down the normal stairs and into the station proper. My route led me to take the slower, unupgraded trains, but those were the more comfortable ones, anyway. As a result, it took several more minutes than the computer had originally anticipated to reach Camber Station.
|
|
||||||
|
|
||||||
My gaze turned wistful at the entrance to access the vacuum train pods. It was a real shame that none of the other tribes cared much for easy transportation between them — but things were getting better, at least between tribes in Farele and in Enigma. Still, none of them would commit to the cost of a vacuum train or even civilian airports, but at least there *were* discussions aimed at improving intertribal transit — the ancient Xunilean bullet trains were starting to show their age. My tribe did what they could to improve performance from our end, but we could only do so much without external funding and support.
|
|
||||||
|
|
||||||
*“Welcome to the Intertribal High-Speed Rail System. This is the Demauge–Xunil Line. Please submit your ticket.”*
|
|
||||||
|
|
||||||
...And they still used those old-fashioned disposable physical tickets, too.
|
|
||||||
|
|
@ -1,27 +0,0 @@
|
|||||||
---
|
|
||||||
title: Axit
|
|
||||||
date: 2020-06-22
|
|
||||||
tags:
|
|
||||||
- barin
|
|
||||||
- unstagnation
|
|
||||||
- literature
|
|
||||||
---
|
|
||||||
|
|
||||||
Imperial Palace\
|
|
||||||
1 Kansei Road\
|
|
||||||
Emina, Asvyn
|
|
||||||
<!-- excerpt -->
|
|
||||||
|
|
||||||
Mr. Brendan May\
|
|
||||||
Chairman\
|
|
||||||
Enigma Alliance\
|
|
||||||
3 Indigo Boulevard\
|
|
||||||
Saiyu, Weilam
|
|
||||||
|
|
||||||
This is to inform the Chairman, in accordance with the Enigma Agreement, adopted at Saiyu on 12 December 1915 ("the Agreement"), that the Asvish Empire intends to exercise its right to withdraw from the Enigma Alliance. Unless the Asvish Empire identifies suitable terms for re-engagement, the Asvish Empire will submit to the Chairman, as per Article 50, paragraph 2 of the Agreement, formal written notification of its withdrawal as soon as it is eligible to do so. Pending the submission of that notification, in the interest of transparency for parties to the Agreement, the Asvish Empire requests that the Chairman inform the parties to the Agreement and the States entitled to become parties to the Agreement of this communication relating to the Agreement.
|
|
||||||
|
|
||||||
Signed,\
|
|
||||||
*Hina Asvyn*\
|
|
||||||
Empress Regnant of Asvyn\
|
|
||||||
22 June 2020
|
|
||||||
|
|
@ -1,76 +0,0 @@
|
|||||||
---
|
|
||||||
title: Complacency
|
|
||||||
date: 2021-05-21
|
|
||||||
tags:
|
|
||||||
- barin
|
|
||||||
- unstagnation
|
|
||||||
- literature
|
|
||||||
---
|
|
||||||
"On the red side we have your ten-year undefeated champion, Ping Pong! And her challenger, Markus Tennis! Will this be the year that the Crowne Cup finally switches hands?"
|
|
||||||
|
|
||||||
<!-- excerpt -->
|
|
||||||
|
|
||||||
The stadium roars as Ping Pong strides over the carpet onto the battlefield. Her stance is ready, eyes drilled into her opponent who merely stares blankly at her. "Let's have a good fight."
|
|
||||||
|
|
||||||
"Agreed." Markus raises his paddle, bouncing on his knees. "Show me what you've got."
|
|
||||||
|
|
||||||
The ball starts on Ping's side. All is quiet on this battlefield except for the clicks of cell phone cameras.
|
|
||||||
|
|
||||||
"Players at the ready!"
|
|
||||||
|
|
||||||
Ping grips the hollow ball a little tighter.
|
|
||||||
|
|
||||||
"Begin!"
|
|
||||||
|
|
||||||
Clack-clack the ball flies off of her hands and a buzzer rings as Ping aces her first serve. Markus looks shocked.
|
|
||||||
|
|
||||||
"Right away Markus is surprised by Ping's masterful serve! It's yet another reminder how she's stayed undefeated for a whole decade!"
|
|
||||||
|
|
||||||
"Well, I have to say, I wasn't expecting that," Markus says sheepishly, scratching the back of his head with his paddle. "You're good."
|
|
||||||
|
|
||||||
Ping stays silent, and lifts the ball up to her paddle again. Markus follows suit and stops talking, his expression changing to one more serious.
|
|
||||||
|
|
||||||
"Ready?"
|
|
||||||
|
|
||||||
"Always have been."
|
|
||||||
|
|
||||||
The ball flies off of Ping's paddle and again and again Markus can never defend against her serves. Sometimes he sends a couple of balls back, but it's never long until he messes up. She wonders how he even made it to the final. As her hands play on autopilot, she glances at the crowd and notes that a good chunk of them have pulled out their phones. Table tennis is mildly interesting at the best of times but now that she's only a couple of points away from the match, 8-0, they all know how the rest of the game is going to go.
|
|
||||||
|
|
||||||
Ping swings her paddle a little too early and Markus scores his first point. He looks like a child who received his first present on Christmas morning. "Ha! How was that?"
|
|
||||||
|
|
||||||
"Very good. But can you keep it up?"
|
|
||||||
|
|
||||||
The audience is suddenly engaged again at the news of a Markus win. Murmurs among them discuss betting odds and the possibility of witnessing the end of an era. Ping smiles inwardly. This is more like it.
|
|
||||||
|
|
||||||
She flubs some more shots and Markus becomes more and more confident, his swings more precise and his reflexes more attentive. As the leading player switches to Markus, the score 9-8, he mocks her. "Sorry to disappoint you, ex-champion. You win some, you lose some, huh?"
|
|
||||||
|
|
||||||
Ping stays calm. She's aware that the crowd is holding their breath. With only two points until Markus wins, and one point until a deuce — Ping Pong has never yet entered a deuce with another player — bets and dreams of making a fortune on her loss fly through the air.
|
|
||||||
|
|
||||||
Ping serves. Her hand moves in slow motion.
|
|
||||||
|
|
||||||
The ball also bounces slowly, and Markus has to lean in to hit it back to her, setting her up perfectly for a smash.
|
|
||||||
|
|
||||||
9-9.
|
|
||||||
|
|
||||||
"Don't get too complacent," she warns.
|
|
||||||
|
|
||||||
"That was a fluke," Markus brushes off, but he's sweating a little more now. "Do that again, can you? I'll be ready for it."
|
|
||||||
|
|
||||||
Ping serves.
|
|
||||||
|
|
||||||
This time, the orange sphere is sent all the way to the edge of Markus's table near the front of the net. He dives, missing it entirely, and ends up prostrating himself in front of her.
|
|
||||||
|
|
||||||
10-9.
|
|
||||||
|
|
||||||
"Get up."
|
|
||||||
|
|
||||||
Markus doesn't say a word as he gets up and goes back to his side. His gamer face is ready, and he claps his cheeks twice. "Is that all you can do?" he manages to say.
|
|
||||||
|
|
||||||
Ping serves.
|
|
||||||
|
|
||||||
The ball blurs, and before Markus can even raise his paddle, it's struck the table once on each side and flown off beyond. An ace.
|
|
||||||
|
|
||||||
"And with a surprise comeback after Markus's own, Ping Pong wins the Crowne Cup for the eleventh year in a row!"
|
|
||||||
|
|
||||||
Ping puts her right palm over her left fist and bows. "You were a fine competitor. I wish you luck on your future ambitions." Without waiting for her opponent's response, she turns around and exits the battlefield, leaving her opponent in the dust.
|
|
||||||
|
|
@ -1,22 +0,0 @@
|
|||||||
---
|
|
||||||
title: Crystal
|
|
||||||
date: 2020-06-13
|
|
||||||
tags:
|
|
||||||
- unstagnation
|
|
||||||
- literature
|
|
||||||
---
|
|
||||||
|
|
||||||
The dark green sun shone on forests of the clearest blue as the rivers of the brightest yellow trickled down the hilly landscape. Unicorn after unicorn pranced alongside the riverbank, frolicking around. I hid in one of the bushes, not daring to take a breath as their playful behaviour brought them closer and closer to my hand.
|
|
||||||
<!-- excerpt -->
|
|
||||||
|
|
||||||
*Just a little more…*
|
|
||||||
|
|
||||||
Right before I reached out to pet what would have been the most luxurious fur ever to be felt by mankind, my dreams were shattered. A sudden weight pressed upon my chest, and pain spasmed throughout my body before I opened my eyes to see my younger brother on my chest, grinning at my suffering.
|
|
||||||
|
|
||||||
“Jordan,” I groaned, slowly laying my head back down on my pillow, “now what did you have to do that for?” I reached for the edge of my blanket, trying to pull it over my head to escape the wrath of the evil sunbeams streaming through my window.
|
|
||||||
|
|
||||||
“Mom says it’s time to get up! She’s making pancakes!” The little rascal jumped up and down before bounding off my bed and out of my bedroom.
|
|
||||||
|
|
||||||
I said sleepily, still not fully awake, “Pancakes…? What are pancakes?”
|
|
||||||
|
|
||||||
Then my brain finally finished booting up. My eyes widened, and I flung my blankets away to finish my morning routine as fast as possible as my nose registered a wonderful aroma floating from the kitchen downstairs. “Pancakes! Wait! Save some for me!”
|
|
@ -1,30 +0,0 @@
|
|||||||
---
|
|
||||||
title: Diplomacy
|
|
||||||
date: 2020-06-29
|
|
||||||
tags:
|
|
||||||
- barin
|
|
||||||
- unstagnation
|
|
||||||
- literature
|
|
||||||
---
|
|
||||||
|
|
||||||
Laveli Guild Headquarters\
|
|
||||||
Laveli Town, Eos
|
|
||||||
<!-- excerpt -->
|
|
||||||
|
|
||||||
Emp. Hina Asvyn\
|
|
||||||
Empress Regnant\
|
|
||||||
Asvish Empire\
|
|
||||||
1 Kansei Road\
|
|
||||||
Emina, Asvyn
|
|
||||||
|
|
||||||
Upon reviewing your first set of proposals regarding Asvyn's withdrawal from the Enigma Alliance ("the Alliance"), Eos would like to raise some concerns in sections 1 (one) and 3 (three).
|
|
||||||
|
|
||||||
- To protect the sovereignty of Eos, Eos cannot permit "all regions of neighbouring Enigma territories within 10 (ten) kilometres of Asvyn" to be ceded to the Asvish Empire. Eos is open to further discussion to clearly define borders.
|
|
||||||
- Additionally, the proposed addition of unified free trade and travel zones mentioned in section 3 (three) is not applicable to section 50 (fifty) of the Enigma Agreement, and any such agreement must be negotiated with the whole of the Enigmatic Council separately.
|
|
||||||
|
|
||||||
Unfortunately, because of the recent formation of the Moyen Channel, Eos has invoked paragraph 51 (fifty-one) in article 2 of the Enigma Agreement, and will be unable to vote on or approve any Level 1 provisions as part of the Enigmatic Council. As a result, any message sent as part of the section 50 (fifty) withdrawal proceedings will not be replied to until 29 June 2022, or when Eos revokes its passage of section 50 (fifty), whichever comes first.
|
|
||||||
|
|
||||||
Signed,\
|
|
||||||
*Retadux*\
|
|
||||||
Ean High Representative to the Enigmatic Council\
|
|
||||||
29 June 2020
|
|
@ -1,73 +0,0 @@
|
|||||||
---
|
|
||||||
title: Divine Assistance
|
|
||||||
date: 2021-05-28
|
|
||||||
tags:
|
|
||||||
- ibia
|
|
||||||
- unstagnation
|
|
||||||
- literature
|
|
||||||
---
|
|
||||||
He took a deep breath.
|
|
||||||
|
|
||||||
And then another one.
|
|
||||||
|
|
||||||
Samuel continued to empty and fill his lungs until his hands stopped shaking.
|
|
||||||
|
|
||||||
Today was the last day.
|
|
||||||
|
|
||||||
<!-- excerpt -->
|
|
||||||
|
|
||||||
The day that their city would be saved, or set for ruin. The council had chosen him to be the one to deliver the message.
|
|
||||||
|
|
||||||
Samuel wrapped his coat tighter around him and firmly shoved the door open with one hand, the other occupied with holding a rolled-up scroll bound with warm red wax. The warm light from the rising sun cast his shadow behind him, as if he was a hero about to set off on his journey.
|
|
||||||
|
|
||||||
The grueling trail to the shrine was covered with snow, and the downpour of snow kept getting in Samuel's eyes, making it near-impossible to see the road in front of him. The only way he was able to tell which way he was slowly trudging through the snow was by following the wide, empty space that was free of the crowded trees typically found in the forest.
|
|
||||||
|
|
||||||
Perhaps she didn't want to be contacted; that was why she had sent this snowstorm to stop him, even though she must have known of the predicament that the city was facing.
|
|
||||||
|
|
||||||
What felt like hours later, as Samuel was wishing again that he had brought a lamp, the constant battering of the hands covering his face suddenly ceased. The crunch of the snow beneath his boots gradually stopped. It became quiet.
|
|
||||||
|
|
||||||
It was still dark outside, the rays from the sun only just peeking over the treetops, unable to penetrate through the dark canopy itself. There was not a chirp from a bird nor a rustle from the wind in the trees to be heard.
|
|
||||||
|
|
||||||
A stone slab surrounded by a large stone arch entered Samuel's view. Two torches to either side of the slab were lit, keeping the stone table warm and dry against the freezing wilderness. Laying ahead of the shrine was a cave, a faint orange glow visible.
|
|
||||||
|
|
||||||
Finally — after so many weeks of fruitless visits, she was finally here.
|
|
||||||
|
|
||||||
Even though he was overwhelmed with relief, Samuel could not help but feel a twinge of frustration at the goddess for waiting until the last day to show up.
|
|
||||||
|
|
||||||
He stepped closer to the mouth of the cave.
|
|
||||||
|
|
||||||
"Who goes there?" The voice beyond the cave was authoritative but neutral. "Halt and state your origin and purpose."
|
|
||||||
|
|
||||||
Samuel closed his eyes and knelt reverently in without moving further in. "O powerful goddess, my name is Samuel, and I come to you from the city of Shope. I beg of you to hear my plea."
|
|
||||||
|
|
||||||
"...Please enter."
|
|
||||||
|
|
||||||
"Thank you, my lady."
|
|
||||||
|
|
||||||
The interior of the circular cavern was warm. A fire in the centre crackled, its shadows along the walls dancing merrily. There was little else in the room aside from its sole occupant sitting cross-legged against the wall, who had clearly been manipulating some string with her hands and now held a complex web in front of her. She stared at Samuel expectantly.
|
|
||||||
|
|
||||||
Her prickling gaze was too much for Samuel and he looked away. In his peripheral vision, he noticed that the woman had returned to tightening and weaving the string with her fingers.
|
|
||||||
|
|
||||||
"So, Samuel, what did you want me to do?"
|
|
||||||
|
|
||||||
"Goddess —"
|
|
||||||
|
|
||||||
"My name is Casia. Use it as you please."
|
|
||||||
|
|
||||||
Samuel hesitated.
|
|
||||||
|
|
||||||
"...Goddess, the city of Shope is under a terrible plight. I humbly ask for your assistance so that the people in the city will live."
|
|
||||||
|
|
||||||
A profound sigh came from the goddess. "What is it this time? Another blight, perhaps? Or have the rats eaten all the grain? Or is it plague — ugh, please don't let it be plagues. I hate plagues, they're so annoying to get rid of. No one ever cleans things properly and the instant I cure you all, someone gets infected again the next day," she grumbled.
|
|
||||||
|
|
||||||
"...I beg your pardon?"
|
|
||||||
|
|
||||||
"Never mind. So what is it?" she repeated, sounding impatient.
|
|
||||||
|
|
||||||
"R-right, yes. My sincerest apologies, my lady," Samuel said hurriedly. "The problem is that the city of Shope is being threatened with utter annihilation."
|
|
||||||
|
|
||||||
The goddess tapped her foot against the ground. "That's not the problem," she said slowly, like she was a junior school teacher explaining something to one of her very dim students. "I would like you to tell me exactly *what* is happening, *why* it's happening, and what you want me to do."
|
|
||||||
|
|
||||||
"How forgiving you are, my lady." The goddess's eye twitched. "Our supply lines are cut off and we are under lockdown. No one can leave or come in. One of your sisters has threatened mass destruction of our unworthy human settlement, so we would humbly ask for your assistance to diffuse the situation."
|
|
||||||
|
|
||||||
Samuel noted with more than a little satisfaction as he bowed that this was the first time in his visit that the goddess looked truly surprised.
|
|
@ -1,25 +0,0 @@
|
|||||||
---
|
|
||||||
title: Dominate
|
|
||||||
date: 2020-07-01
|
|
||||||
tags:
|
|
||||||
- barin
|
|
||||||
- unstagnation
|
|
||||||
- literature
|
|
||||||
---
|
|
||||||
|
|
||||||
A huge uproar erupts from the crowds of Saiyu as the results of the 2020 Weilamese election are revealed to the world.
|
|
||||||
<!-- excerpt -->
|
|
||||||
|
|
||||||
"…and your new President of Weilam until 2024 is…Roy Tamino!"
|
|
||||||
|
|
||||||
"What the hell!" One citizen has his mouth hanging open. "How—how did *he* win!"
|
|
||||||
|
|
||||||
A few metres away, another is waving a campaign poster in the air, cheering wildly for her preferred candidate. "Woo! For Weilam! Young power!"
|
|
||||||
|
|
||||||
Standing off to the side is a supporter of a third party candidate, crying with his face in his hands.
|
|
||||||
|
|
||||||
Under the shadow of a building, a group of people chant with masks. "Axit! Axit! Axit!"
|
|
||||||
|
|
||||||
The candidate of the other party is shaking hands with the president-elect, a forced smile on his face. "Congratulations, Roy! This result was unexpected to me too, don't worry!"
|
|
||||||
|
|
||||||
Roy Tamino himself is staring at the votes on the screen, seemingly oblivious to his surroundings. "But—I never wanted this," he whispers. "I'm just nine years old; this was just a joke…Do *I* have to deal with Farele and Enigma?"
|
|
@ -1,33 +0,0 @@
|
|||||||
---
|
|
||||||
title: Father and Daughter
|
|
||||||
date: 2020-07-02
|
|
||||||
tags:
|
|
||||||
- barin
|
|
||||||
- unstagnation
|
|
||||||
- literature
|
|
||||||
---
|
|
||||||
|
|
||||||
Trumpets blare around the royal procession as Princess Dazel bows down in front of her father.
|
|
||||||
<!-- excerpt -->
|
|
||||||
|
|
||||||
"My daughter, what news have you brought for us today?" King Rambel Bheosetrawpe Mydrule leers imposingly in his startling green robes, staring down at the princess.
|
|
||||||
|
|
||||||
"Father, I have returned from the Waterfall of Fate, but…" Dazel lowers her head further, "I could not awaken my power."
|
|
||||||
|
|
||||||
The king lets out a long sigh, mouth set in a firm line, then places his hand on his daughter's shoulder, beckoning for her to stand up. "Rise, Dazel. We shall discuss this matter further in a more…secluded area."
|
|
||||||
|
|
||||||
Dazel somewhat fearfully stands, but she follows her father behind the throne, away from any prying eyes. King Rambel pushes the heavy wooden doors together behind her. Once he verifies that there is no one else to overhear their discussion, he kneels, placing a hand on Dazel's shoulder once again. He opens his mouth to speak, but the princess starts speaking rapidly before words can form.
|
|
||||||
|
|
||||||
"I'm sorry, father, but I *can't* do this! I've spent my whole childhood praying and praying! Please, please let me do the things I love! I can't do this anymore—"
|
|
||||||
|
|
||||||
Rambel raises a hand for silence. "Dazel, I understand—"
|
|
||||||
|
|
||||||
"No, you don't!"
|
|
||||||
|
|
||||||
"Do not interrupt me. As I was saying, I understand your situation. And you are correct. I have been far too harsh on you ever since your mother passed away. I have pushed you to awaken your gift. But I now see the error of my ways." He stands, looking directly into her tearful eyes. "You may go. Live your life, and may it be happier than what I have put you through."
|
|
||||||
|
|
||||||
The princess looks skeptical, but also hopeful. "Do you mean that, father?"
|
|
||||||
|
|
||||||
The king belts out a hearty laugh and slaps her on the cheek. "Ha, you believed me for a moment there! Of course not," he snorts, "now you must head to the Forest of Destiny."
|
|
||||||
|
|
||||||
Dazel just looks broken and crestfallen as she turns away.
|
|
@ -1,33 +0,0 @@
|
|||||||
---
|
|
||||||
title: Growing Pains
|
|
||||||
date: 2021-05-25
|
|
||||||
tags:
|
|
||||||
- barin
|
|
||||||
- unstagnation
|
|
||||||
- literature
|
|
||||||
---
|
|
||||||
Renge Academy\
|
|
||||||
No address\
|
|
||||||
Biyori, Asvyn
|
|
||||||
|
|
||||||
<!-- excerpt -->
|
|
||||||
|
|
||||||
Hina Asvyn\
|
|
||||||
Empress\
|
|
||||||
1 Kansei Road\
|
|
||||||
Emina, Asvyn
|
|
||||||
|
|
||||||
Empress,
|
|
||||||
|
|
||||||
With all due respect, as one of Your Majesty's principal advisors, the mass annexation of Enigmatic territories is not only in grave violation of Section 2 of the Enigma Agreement, but has also led to Empire forces being stretched far too thin across the nation. The Principal of Intelligence informs me that they are unable to conduct adequate surveillance to prevent uprising. Combined with the Favonius resistance and ongoing struggle against Asvish control from the previously Enigmatic territories, I urge you to reconsider the attack on further Enigmatic territories lest they fully commit to war — a war we will be unable to defend against.
|
|
||||||
|
|
||||||
I send this letter to you from the Renge Academy in Biyori, one of the few newest regions taken from the Enigma Alliance. Empire officials are few and far between, and it is only because of the lethargic nature of the citizens here that this region is currently stable.
|
|
||||||
|
|
||||||
To quell the rebellion and restore order to the Empire, I would like to put forth my agreement for the suggestion of the Principal of Continental Security to accept aid offered by the Farelean nation of Ciers. No matter how it might appear on the world stage, Empress, the domestic situation is truly dire and the Empire is at serious risk of becoming fractured. Although not my place to say, I would place under consideration that building a positive relationship with other nations would be beneficial to Asvyn's continued survival after the impending withdrawal from the Enigma Alliance.
|
|
||||||
|
|
||||||
I shall be visiting and examining the statuses of the far more conflicted regions of Sayoasa, Yuru, and Aineiru in the upcoming weeks. In order to make a proper assessment, I request for a small dispatch of bodyguards in the event of chaos.
|
|
||||||
|
|
||||||
Yours,\
|
|
||||||
Kuvira Smith\
|
|
||||||
Principal for Homeland Security\
|
|
||||||
25 May 2021
|
|
@ -1,54 +0,0 @@
|
|||||||
---
|
|
||||||
title: Honour
|
|
||||||
date: 2020-07-06
|
|
||||||
tags:
|
|
||||||
- unstagnation
|
|
||||||
- literature
|
|
||||||
---
|
|
||||||
|
|
||||||
"Arro, since you're going to be the saviour of our world, you just have to know one thing. Under *no* circumstance will you reveal your abilities. Even though you held a *press conference* yesterday, there's still a chance we can keep you hidden a little longer. Is that clear?"
|
|
||||||
<!-- excerpt -->
|
|
||||||
|
|
||||||
Arro nodded his head quickly. "Yes, sir. I'll make sure I only control one element in front of people."
|
|
||||||
|
|
||||||
"Good child," his master said, patting his head. "You understand what must be done."
|
|
||||||
|
|
||||||
------
|
|
||||||
|
|
||||||
"Welcome to the 2020 Kolaltan Magic Championship! We're going to start off with—on my left over here—the Groundhogs versus—on my right—the Electric Boogaloos!" The announcer gestured wildly at the two teams of five heading from opposite ends of the arena. The crowd cheered exuberantly. Arro, as the leader of the Electric Boogaloos, confidently smirked at the Groundhogs.
|
|
||||||
|
|
||||||
"Groundhogs, eh? More like you're-going-*down*-hogs, am I right?"
|
|
||||||
|
|
||||||
There was a quiet groan behind Arro. The Groundhogs just looked at the Boogaloos oddly, and their leader shook her head. "Was that supposed to be a roast?"
|
|
||||||
|
|
||||||
Arro frowned for just a second when he realised that his joke was not well-received. "No, of course not. I was just…testing my vocal chords." He bit his tongue before he could manage to insult himself further. "Anyway, you're going *down!"*
|
|
||||||
|
|
||||||
"The Boogaloos have been absolutely *dominating* the tournament so far, using their spectacular electric zappy powers to ruin everyone else's days!" the announcer boomed. "But now they face an opponent with earth powers! And everyone knows that electricity is completely ineffective against the ground! Whatever will they do!"
|
|
||||||
|
|
||||||
"Yeah, electric isn't a good element to pick, you know," one of the Groundhogs pointed out.
|
|
||||||
|
|
||||||
"Shut up! We got this, so you guys prepare to…uh, wet your shirts!"
|
|
||||||
|
|
||||||
There was silence.
|
|
||||||
|
|
||||||
There was a cough in the crowd.
|
|
||||||
|
|
||||||
"…Well, I'm sure the Boogaloos' magic is better than their trash-talking, so why don't we get started? Players, are you ready? Go!"
|
|
||||||
|
|
||||||
Immediately after the whistle was blown to denote the start of the match, Arro's team fired lightning bolt after lightning bolt. One of the Groundhogs was too slow and subsequently convulsed out of the ring, but the others raised shields made out of earth. Rocks and dirt were flung in the direction of the Boogaloos, absorbing any negative charge that might have reached the opposing team. One by one, the Groundhogs used their element to strike down Boogaloo after Boogaloo.
|
|
||||||
|
|
||||||
At last, only Arro was left, throwing arcs of electricity in the hopes of randomly breaching the earthen barrier of the Groundhogs.
|
|
||||||
|
|
||||||
"Give up, man," said a Groundhog. "You can't win."
|
|
||||||
|
|
||||||
*My honour,* thought Arro. *It* cannot *take another beating. I can win. I must win. I will win. I will not lose my honour!*
|
|
||||||
|
|
||||||
And a wave of water streamed from his fingertips, crashing into his opponents. Three of them fell off the edge, surprised by the sudden onslaught of their weak element. The crowd roared with approval.
|
|
||||||
|
|
||||||
"What a surprise!" said the announcer, sounding very surprised indeed. "It turns out that the Electric Boogaloos have an Elemental Master on their team! And from what we can see, he *demolishes* the Groundhogs using his water powers, *sweeping* the team! It's one-on-one, folks, and I don't see how the last Groundhog is going to—"
|
|
||||||
|
|
||||||
Suddenly, a giant fist erupted from the ground, punching Arro out of the ring instantly.
|
|
||||||
|
|
||||||
"…win," the announcer finished lamely. "And the Groundhogs win with that surprise attack! From what we can see, that attack must have completely drained its caster…"
|
|
||||||
|
|
||||||
Arro spat out water as he tread up to the surface. He lay on his back, looking at the ceiling. *I failed. I must redeem myself. I must regain my honour.* His eyes flickered over to the last Groundhog, who had his fist in the air in triumph. *I must have my revenge.*
|
|
@ -1,23 +0,0 @@
|
|||||||
---
|
|
||||||
title: How the Moyen Channel was Formed
|
|
||||||
date: 2020-06-23
|
|
||||||
tags:
|
|
||||||
- barin
|
|
||||||
- unstagnation
|
|
||||||
- literature
|
|
||||||
---
|
|
||||||
|
|
||||||
"Good morning, citizens of Ptuyo!"
|
|
||||||
<!-- excerpt -->
|
|
||||||
|
|
||||||
My phone's screen flickers once as the video I'm watching is replaced by the face of a young man with glasses, luxurious golden locks, and an exquisite moustache: Riley, Director of Public Affairs in our tribe. "I do hope you're all having a great day so far," he says excitedly, "but I'm afraid I must interrupt you for an important message from our Supreme Leader, Sebastian."
|
|
||||||
|
|
||||||
Riley's face vanishes from my phone, and I take a moment to look around at everyone else. They're attached to their phones, too. Sebastian's stubbled face appears on all our displays. His grin is enthusiastic, and he spreads his hands at the camera before speaking.
|
|
||||||
|
|
||||||
"Thank you, Riley! I know you're all doing really important things, but I'm afraid that I've got really important news! Right after this message from our Director of Defense, it's the one and only…Crow!"
|
|
||||||
|
|
||||||
The image on the screen flashes one more time to show someone who looks somewhat to a younger, malnourished Riley. Crow has glasses, but unlike Riley, he's distinctly more…frail. His black hair is disheveled, but I know that his endless patience and big brain makes him invaluable to our tribe.
|
|
||||||
|
|
||||||
"Yes! Guys, do you know what we just did? Do you know we just did?" Crow is sitting in his office, wearing a simple maroon t-shirt. His smile is infectious, and I'm all ears, ready to hear what is about to come next. Any de-escalation of the rising tensions between Preton and Farele would be sorely welcome, as I wouldn't want to fight—
|
|
||||||
|
|
||||||
"We broke the world — with just a single bomb!"
|
|
@ -1,15 +0,0 @@
|
|||||||
---
|
|
||||||
title: Injustice
|
|
||||||
date: 2020-06-25
|
|
||||||
tags:
|
|
||||||
- barin
|
|
||||||
- unstagnation
|
|
||||||
- literature
|
|
||||||
---
|
|
||||||
|
|
||||||
It is — unethical and *deeply* immoral to block our people from knowledge. Is Leeco not a free tribe? Do we not accept those in pursuit of information? We cannot block our citizens from learning more about the universe to further the human race. Our tribe was founded on the principle of helping each other learn and grow by education. We cannot learn if we never challenge our beliefs, no matter how deep their roots lie — you might remember how ingrained racism was in Leeco so many decades back — and we cannot grow as a society if we resist change! If we do not rapidly adapt to the world, the world will rapidly adapt around us. And that is unacceptable.
|
|
||||||
<!-- excerpt -->
|
|
||||||
|
|
||||||
In order to face the changing world, we must cease our aimless — no, *destructive* actions of what can only be described as censorship — and allow Leecans to learn and share their knowledge of any topic they wish to! It is not the duty of that government to restrict what art or science one learns. It is not the responsibility of the government to hold still our way of life. The *people* influence the government, certainly not the other way around. To claim so is to be utterly incorrect in a free and just tribe.
|
|
||||||
|
|
||||||
—George Anstion, assassinated five days before the Koran Revolution.
|
|
@ -1,39 +0,0 @@
|
|||||||
---
|
|
||||||
title: java.lang.NullPointerException
|
|
||||||
date: 2020-06-19
|
|
||||||
tags:
|
|
||||||
- barin
|
|
||||||
- unstagnation
|
|
||||||
- literature
|
|
||||||
---
|
|
||||||
|
|
||||||
*Test 19/20 failed: Memory access violation.*
|
|
||||||
|
|
||||||
I let out a loud exhale as my fingers twitched in front of the keyboard. Someone else walked by.
|
|
||||||
<!-- excerpt -->
|
|
||||||
|
|
||||||
“Um, Siava, are you all right?”
|
|
||||||
|
|
||||||
I fought to keep my hands under control as they strained for something, anything to latch onto and ruin. *Bad hands. Do not strangle her. She is your friend. Friends are good. Friends are helpful. Friends are calming.*
|
|
||||||
|
|
||||||
“Ah, Selene! No, everything is perfectly fine! It’s only the *tenth time that* this *stupid* program *breaks* on me and I don’t know *why* but there is absolutely nothing wrong whatsoever! Whichever garbage Xunilean engineer designed this stupid framework needs to go to hell but I’m fine! Perfectly fine and sane and sane and fine!”
|
|
||||||
|
|
||||||
Selene looked concernedly from my twitchy fingers, which were now gripping the keyboard tightly — no, *bad hands*, don’t break the keyboard — to my beaming face. “Yeah, no. You’re about to blow up. Come get a drink with me?”
|
|
||||||
|
|
||||||
I shook my head, returning to my computer to hammer away lines upon lines of troubleshooting code with the strength of determination and coffee. “You don’t understand. This is going to work. All I need is *one more* try! I’ve spent hours on this, I’m sure I can fix it I know exactly what went wrong I’m *going to finish it tonight—*”
|
|
||||||
|
|
||||||
My monitor suddenly went black. I turned to see Selene moving her hand away from the power button of the workstation. The fury of a thousand suns burned within me, and adrenaline pumped throughout my body, demanding I settle the matter immediately with the one who *dared* take me away from my work. I stood up and glared at my ex-friend. “Selene.”
|
|
||||||
|
|
||||||
She put her hand on my shoulder, applying just enough pressure to turn me in the direction of the break room. “Siava. Look, I’m sorry, but it’s past midnight! The buses and subways aren’t running today, so you’re my carpool home, and everyone else has gone already!” She gestured at the rest of the darkened cubicles.
|
|
||||||
|
|
||||||
“That does not mean you get to take me away from my work,” *you traitorous woman,* I added mentally. “I only needed a couple more minutes.”
|
|
||||||
|
|
||||||
“Calm down,” Selene said soothingly. Only now did I notice that she had already packed up all my things — maybe she wasn’t the worst person in the world after all. “Even if you spent hours more, there’s no guarantee you can finish before daybreak,” she yawned, “and I’d like to get at least six hours of sleep tonight. So we’re leaving, right now.”
|
|
||||||
|
|
||||||
Now that I had stopped looking at that accursed screen, my blood pressure returned to slightly more regular levels. I sighed. “You’re right, sorry. I guess I was just a little worked up.”
|
|
||||||
|
|
||||||
I started picking up my things that Selene had very nicely finished packing up for me. She smiled. “That’s the spirit. There’s no need to worry about anything. It’s a good idea sometimes to come back to something later, after you’ve had a break.”
|
|
||||||
|
|
||||||
We were already in my car and on the highway when she asked, “You did save your work before I turned your computer off, right?”
|
|
||||||
|
|
||||||
As my car headed down the ramp off the high-speed road, any onlooker would only see one person in the vehicle.
|
|
@ -1,56 +0,0 @@
|
|||||||
---
|
|
||||||
title: Kuro in Baccalor
|
|
||||||
date: 2021-08-19
|
|
||||||
tags:
|
|
||||||
- ibia
|
|
||||||
- unstagnation
|
|
||||||
- literature
|
|
||||||
---
|
|
||||||
"…And never come back again, boy!"
|
|
||||||
|
|
||||||
You fall to the ground with an *oof!* as the bartender tosses you out of the tavern. Scowling, you yell behind his retreating back, "You're wrong! All of the goddess cities are wrong! The only way for people like us to prosper is to take control of our own lives!"
|
|
||||||
|
|
||||||
<!-- excerpt -->
|
|
||||||
|
|
||||||
It's been only a few hours since you entered the goddess city of Baccalor, but since then you've been forcibly removed from nearly every business you've gone into. How incredibly rude — but to be expected of a goddess city, filled with entitled, lazy sheep who refuse to look ahead past the blindfold in front of their faces.
|
|
||||||
|
|
||||||
The symbol of the goddess cities on the tavern sign, a heptagon inscribed in another heptagon, cowers before your knowledge.
|
|
||||||
|
|
||||||
Brushing some dirt off from your rear, you think that the library across the street must contain people who are even *somewhat* more enlightened than the women — with the occasional man — drinking their problems away.
|
|
||||||
|
|
||||||
"Welcome!" a young woman greets you warmly with a smile as the bell above the door rings to signify your arrival in the library. It's only natural that she would.
|
|
||||||
|
|
||||||
You nod politely in return, attention stolen by the rows upon rows of bookshelves that fill both floors of the building. There are many more books in Baccalor's library than in your hometown of Stuco's. But of course, unlike Stuco, you scoff as you skim the titles across each shelf, goddess cities don't curate their selection and so the junk such as the (false!) *glorious history* of the goddesses lies on the wooden racks.
|
|
||||||
|
|
||||||
The book is worn, well-read. It appears that the disease that is worship runs much deeper in this city than you had imagined. You'll need to work harder if you want to show them the truth.
|
|
||||||
|
|
||||||
Strutting up to the nearest group of women reading at the long wooden desk — seriously, where did all the men in the library go? — and clear your throat to announce your presence. Only a few raise their attention from their books.
|
|
||||||
|
|
||||||
"Yes?" asks a rich woman with glasses. "What do you want?"
|
|
||||||
|
|
||||||
You have to admit that less than half of the people there giving you attention is a little disheartening. "Ah — I'd just like to ask you all if you knew the goddesses' role in the destruction of Malum?"
|
|
||||||
|
|
||||||
Collective exasperated sighs come from all around you, even from those who weren't looking at you.
|
|
||||||
|
|
||||||
"Look, child," says the rich woman again, "How old are you, exactly?"
|
|
||||||
|
|
||||||
Ad hominem. A classic diversion tactic. You'd never fall for it —
|
|
||||||
|
|
||||||
But she questioned your age! Really, even as a teenager, you'd know way more than these Baccalorean snubs.
|
|
||||||
|
|
||||||
"I'm fourteen," you declare proudly.
|
|
||||||
|
|
||||||
The woman places her open book face-down, resting her elbows on the table with clasped hands. "Please understand," she says firmly, "that Malum isn't real, no matter what Stuco always says. Historians from across the six goddess cities have investigated our history and found no evidence of another goddess city. There are only six goddesses, anyway, so who would rule over this supposed Malum?"
|
|
||||||
|
|
||||||
The clear disrespect from the uneducated women is really testing your limits. You can accept blissful ignorance, yes, but to actively defend lies even when the truth is right in front of them? Utterly unacceptable.
|
|
||||||
|
|
||||||
"Lady," you don't quite mean to but your tone comes out a bit harsher than you'd like. "I know you've all been brainwashed by your so-called patron goddess, but think with your own heads for a moment, okay?" You spread your hands as if lecturing a child. "That's what the goddesses want you to think. They have you all wrapped along their little finger. They just want to control us people so they can exploit us! They just want to —"
|
|
||||||
|
|
||||||
A finger taps your shoulder, interrupting your tirade. You whirl around to see who dared to interrupt the truth.
|
|
||||||
|
|
||||||
"Excuse me." The librarian who greeted you earlier still smiles as her voice turns hard. "I'm afraid I'll have to ask you to leave. You're disturbing our guests."
|
|
||||||
|
|
||||||
The rich woman shakes her head as if she thinks she's so much smarter than you. "You'll understand when you're older," she says off-handedly, returning to her book. The rest follow.
|
|
||||||
|
|
||||||
As you're led by the shoulder to the exit, you call back, "When the goddesses take all your crops and force you to kill, don't say I didn't tell you so!"
|
|
||||||
|
|
@ -1,35 +0,0 @@
|
|||||||
---
|
|
||||||
title: Leadership
|
|
||||||
date: 2020-07-22
|
|
||||||
tags:
|
|
||||||
- barin
|
|
||||||
- unstagnation
|
|
||||||
- literature
|
|
||||||
---
|
|
||||||
|
|
||||||
"…preserve, protect, and defend the Constitution of Weilam."
|
|
||||||
<!-- excerpt -->
|
|
||||||
|
|
||||||
"…preserve, protect, and defend the Constitution of Weilam," I repeated.
|
|
||||||
|
|
||||||
"Congratulations, Mr. President," said the Chief Justice. I nodded, raising my hand above my head to take the taller man's hand. As we were released from the handshake, I took my other hand and waved at the crowd, who burst into cheers.
|
|
||||||
|
|
||||||
Standing off to the side, my parents tearfully clutched each other tightly, their faces smiling but white.
|
|
||||||
|
|
||||||
My friends from school had gathered on the sidewalk. I thought I heard them cheering the loudest.
|
|
||||||
|
|
||||||
------
|
|
||||||
|
|
||||||
"Mr. President, on your first day in office, I would recommend starting off with enacting the first item on your agenda: allowances for every citizen."
|
|
||||||
|
|
||||||
"Okay! You can do that, right? Can I go visit my friends now?" Honestly, all these fancy people wanted to talk to me, and it was getting annoying. There were people congratulating me, people asking me for leadership, people asking me for help—a twelve-year-old only has so much time, you know—I just wanted a day off.
|
|
||||||
|
|
||||||
"Sir, you promised the people you would enact change, so by not doing anything productive, you're going to make everyone doubt you." My advisor knew well, of course, but the people had elected *me*. No matter that he was nearly four times my age, *I* was the one in charge.
|
|
||||||
|
|
||||||
"Absolutely not, George. A *man*," I noticed gleefully that my advisor flushed at the emphasis of that word, "needs to manage and balance his time wisely. And I haven't been doing that lately. We can work on changing the world tomorrow."
|
|
||||||
|
|
||||||
"I'll call your mother and tell her that you haven't been doing your job properly," he threatened.
|
|
||||||
|
|
||||||
My face paled. "No! Not mom! I'll do anything! I'll sign everything! I'll fill out all the forms! I would write law stuff but I don't know how to do that! I'll *even draw you a butt!"*
|
|
||||||
|
|
||||||
He smiled. "That is appreciated, but not at all necessary. Now, son, why don't we get you over to the Chamber of Representatives…"
|
|
@ -1,44 +0,0 @@
|
|||||||
---
|
|
||||||
title: Magic Paint Gun Cartridges
|
|
||||||
date: 2021-05-31
|
|
||||||
tags:
|
|
||||||
- unstagnation
|
|
||||||
- literature
|
|
||||||
---
|
|
||||||
*Pew pew!*
|
|
||||||
|
|
||||||
Splotches of colour cover the previously white wall until a coherent picture forms.
|
|
||||||
|
|
||||||
<!-- excerpt -->
|
|
||||||
|
|
||||||
"Success!" Minestro steps back from his magic paint gun to admire his freshly painted Nyan Cat. Illuminated by harsh fluorescent lighting, to either side of the pixel art cat and stretching for many metres are recreations of other memes. To the right of the paint gun lies a stack of papers whose contents have already been scanned and blasted on the walls.
|
|
||||||
|
|
||||||
Minestro takes the last picture to the left of the paint gun mounted on his table and feeds it into a scanner sitting beside it. "Last one and that'll be it for the day."
|
|
||||||
|
|
||||||
As soon as the scanner spits the picture back out at him, the gun beeps angrily. Reading the text with a frown, Minestro curses the fallacy of printers of any kind still being utterly useless even with modern technology and magic.
|
|
||||||
|
|
||||||
OUT OF INK.
|
|
||||||
|
|
||||||
The regret of choosing this model of magic paint gun has more than caught up to him already. Supposedly able to infinitely use a single cartridge, it had turned out that the cartridge would empty as soon as the paint gun stopped drawing from it.
|
|
||||||
|
|
||||||
Minestro sighs. The paint gun has analysed the picture and provided him with a colour map, but it can't tell him how many cartridges he needs to buy — it just gives an "recommended estimate" of a hundred cartridges of each colour. Since the printer moves from left to right, top to bottom like a book, he'll need to calculate that number himself if he doesn't want to be scammed by Big Printer.
|
|
||||||
|
|
||||||
Useless machine.
|
|
||||||
|
|
||||||
Since some colours are made from a mix of other colours, Minestro reasons, he can use just one cartridge if a colour before or after also uses the same base colour. Luckily, it's not terribly difficult, and he's able to crunch the numbers in his head. The end result comes out to ten red cartridges, thirteen yellow cartridges, and four blue cartridges.
|
|
||||||
|
|
||||||
One trip to the hardware store later, Minestro slots in his third-party ink into the gun, ignoring any warnings about warranty or damage to the gun and lets it rip.
|
|
||||||
|
|
||||||
Minestro decides that the resulting image is beautiful and well worth the investment of both the paint gun and the shopping trip.
|
|
||||||
```
|
|
||||||
.B................
|
|
||||||
..YY...........YYY
|
|
||||||
...YYY.....YYYY...
|
|
||||||
..YYYYYYYYYYYYY...
|
|
||||||
.YYBBYYYYYBBYYY...
|
|
||||||
.RRYYYYBYYYYRRY...
|
|
||||||
.RRYYYOOOYYYRRY...
|
|
||||||
..YYYYOOOYYYYYY...
|
|
||||||
.YYYYYYYYYYYYYY...
|
|
||||||
```
|
|
||||||
|
|
@ -1,32 +0,0 @@
|
|||||||
---
|
|
||||||
title: Nora
|
|
||||||
date: 2021-05-23
|
|
||||||
tags:
|
|
||||||
- unstagnation
|
|
||||||
- literature
|
|
||||||
---
|
|
||||||
Long ago, the gods wiped themselves out in a magical war of epic proportions, leaving the world in ruins. The remnants of the forces escaped to the stars and skies above, but the carnage had lasting effects on Earth. The age of the gods was over.
|
|
||||||
|
|
||||||
<!-- excerpt -->
|
|
||||||
|
|
||||||
Many millennia later, the very first animals appeared, along with humans. They built a civilisation with elemental skills granted to them by the latent energies in the ground but attributed to gods in the sky. Some were able to control the flow of the rivers. Some were able to breathe life into flames. Others still had the ability to raise the earth. Yet most were powerless against the beast animals that attacked the people.
|
|
||||||
|
|
||||||
Animals were not the only threat to society. The powers used to construct could also be used to destroy. One being was known to cause much strife to those who lived in the cities. Said to be a powerful figure corrupted by power, all conflicts between the people paled in comparison to this figure. Against the Demon Lord, even the most stubborn of enemies would band together to fight a new common enemy.
|
|
||||||
|
|
||||||
It would never succeed, until...
|
|
||||||
|
|
||||||
At last, a white-haired boy ended it all. The chosen one himself, he did so with nothing but a ragtag band of elementals and friendship and determination. He discovered the secrets of the world and the true story behind the gods that were worshipped around the continent.
|
|
||||||
|
|
||||||
He kept moving forward, forging his own path against the trials and tribulation set against him.
|
|
||||||
|
|
||||||
He came to trust his friends , protecting and letting them protect them in the face of unimaginable danger.
|
|
||||||
|
|
||||||
He learned much on his journey, growing into a strong and compassionate man.
|
|
||||||
|
|
||||||
He and I...
|
|
||||||
|
|
||||||
The domain of the gods is ever so lonely.
|
|
||||||
|
|
||||||
<hr>
|
|
||||||
|
|
||||||
This Unstagnation short was heavily influenced by one of the works of Nettlespike.
|
|
@ -1,78 +0,0 @@
|
|||||||
---
|
|
||||||
title: Patron
|
|
||||||
date: 2021-05-30
|
|
||||||
tags:
|
|
||||||
- ibia
|
|
||||||
- unstagnation
|
|
||||||
- literature
|
|
||||||
---
|
|
||||||
"Please hurry, goddess."
|
|
||||||
|
|
||||||
What was this human thinking, pushing her around to "hurry"? She was one of the seven goddesses of the world! Even if she couldn't smite him on the spot, there were at least five ways she could think of off the top of her head to torture him until he begged her for mercy.
|
|
||||||
|
|
||||||
<!-- excerpt -->
|
|
||||||
|
|
||||||
"Was it Kemia? Histia? Tell me exactly what my sister said," she demanded as she followed the running Samuel through the snowy forest.
|
|
||||||
|
|
||||||
"*Huff*…it was Mafia. She said that…*puff*…she was bored and was going to kill us all," Samuel wheezed.
|
|
||||||
|
|
||||||
"Mafia..." Casia mumbled. "I see. I'll help you, then. Leave her to me."
|
|
||||||
|
|
||||||
---
|
|
||||||
|
|
||||||
The midday sun reflected off of the snow compressed by the hundreds of frantic footsteps of the citizens of the city of Shope.
|
|
||||||
|
|
||||||
"She has arrived with her ultimatum," Samuel observed solemnly as they stepped through the gates. "We now entrust our lives to you, Lady Casia."
|
|
||||||
|
|
||||||
"Like I said, my name is Casia. Just Casia." Mortals and their dim-wittedness never failed to impress her with their terrible memories and comprehension abilities. "Don't worry about Mafia," she said, waving at a young boy glimpsing at them through a gap in a window. "Just make sure none of you people get in my way."
|
|
||||||
|
|
||||||
Samuel bowed as they reached the town square. "As you wish,." He stepped ahead of her toward a group of old men in heavy jackets who were gesturing wildly at a bored-looking young woman sitting cross-legged on the rim of the frozen fountain, her hands casually placed behind her to support her position.
|
|
||||||
|
|
||||||
The woman looked up at their arrival and beamed upon catching Casia's eye, waving cheerfully. "Hey, Cassie! How're you doing?"
|
|
||||||
|
|
||||||
Samuel excused himself and the old men and huddled them together, whispering rapidly. Casia put her hands on her hips and frowned at the woman. "Mafia, what's happening? Some mortal complained to me about some threat you made to destroy all their food or something."
|
|
||||||
|
|
||||||
Mafia laughed, waving the matter off with a hand. "Oh, that? I was just having some fun. Even if we can't control mortal humans directly, that just makes it a little more challenging, you know?"
|
|
||||||
|
|
||||||
Casia was not amused. "What game?"
|
|
||||||
|
|
||||||
Leaning forward and resting her chin on the backs of her hands, Mafia smiled coldly. "Don't you ever want to see how they'll react when you make all of their food rotten? Maybe they'll fight among each other, breaking out in civil war," she gushed. "Their city's no doubt going to crumble as they know it, but think about how they might run to other settlements, how the children will be orphaned, or even how the people are slowly going to lose hope and give into despair! How much stress can society take before it shatters? It's just so much more interesting when you can see exactly what every person's thinking!"
|
|
||||||
|
|
||||||
Casia was silent for a few moments. It did seem like a good experiment — for sure Fisia and Kemia would agree without hesitation. However... She looked back at Samuel, who wiped his brow as he scribbled over pieces of paper and handed them out to the elders, pointing them in every direction.
|
|
||||||
|
|
||||||
What he had said on their multi-hour run back to Shope about each person in the city...
|
|
||||||
|
|
||||||
From the various buildings facing the town square, she could feel the gazes of dozens of the people who lived in their village, each with their own aspirations and hopes.
|
|
||||||
|
|
||||||
The boy on the second floor of the barber's shop, who wanted to get better at his craft and cut his own hair so that he might be able to spend more time with the girl he liked whenever she came to get a haircut — how foolish.
|
|
||||||
|
|
||||||
The girl training to take over her parents' apothecary, constantly lathering products in her hair in the hopes that it might grow faster and so she might be able to visit her friend at the barber's more often — doubly foolish.
|
|
||||||
|
|
||||||
The boy and the girl's parents, who looked on at the obvious schemes of their children with amusement but encouragement — it was very irresponsible of them.
|
|
||||||
|
|
||||||
Their futures now depended on her actions today.
|
|
||||||
|
|
||||||
"Mafia, I'm afraid that I can't support this. Please do not interfere with the matters of Shope from now on. I'll revert anything you try to do."
|
|
||||||
|
|
||||||
"You're no fun," Mafia sighed, brushing the snow off of her pants as she stood and turned away from her. "I don't see why you care so much about mortals. Whatever. I guess I'll just come back when you're not looking." She winked at Casia.
|
|
||||||
|
|
||||||
"That...will never happen!" Casia called after her sister. "I'll stay here the whole time and protect this city! I'll watch over it to make sure you or anyone else lays a finger on it!"
|
|
||||||
|
|
||||||
That stopped Mafia from walking out of the city. "Oh, really?" She smirked. "You're going to chain yourself to these mortals? I wonder how long it'll take before you get bored too."
|
|
||||||
|
|
||||||
Casia glanced at the faces in each home that regarded their conversation.
|
|
||||||
|
|
||||||
"I know you very well, Cassie. In a few thousand years, you're going to grow bored. I'll keep an eye on this place for when that happens.."
|
|
||||||
|
|
||||||
"I will not!"
|
|
||||||
|
|
||||||
"Whatever you say, sister. See you then!" Mafia strode off. None of the elders or Samuel dared to stop her.
|
|
||||||
|
|
||||||
After Samuel made sure that Mafia had exited the city, he raised his open palm in the air, and then slowly closed it.
|
|
||||||
|
|
||||||
Cheers erupted around the city as people swarmed out of the homes and shops. Samuel himself held his clenched fist up in the sky triumphantly for a few more moments before he joined Casia by the frozen fountain.
|
|
||||||
|
|
||||||
"Thank you for your assistance. We will never forget our debt to you," his eyes were shining as he and the elders behind him kneeled in front of her, "our patron goddess. We must construct a shrine! Let this day be marked as the Day of the Goddess — a day of celebration and offerings as a reminder to the benevolence of our divine leader!"
|
|
||||||
|
|
||||||
Casia let out a small smile as she nodded firmly at Samuel's proposition. With offerings and a city of citizens in her debt, who could grow bored?
|
|
||||||
|
|
@ -1,20 +0,0 @@
|
|||||||
---
|
|
||||||
title: Re-education
|
|
||||||
date: 2020-06-30
|
|
||||||
tags:
|
|
||||||
- barin
|
|
||||||
- unstagnation
|
|
||||||
- literature
|
|
||||||
---
|
|
||||||
Welcome to Herdit's Social Education Course! As the successful completion of this course is compulsory for acquiring citizenship in Herdit, we hope you learn from your experience in this program.
|
|
||||||
<!-- excerpt -->
|
|
||||||
|
|
||||||
When you are admitted into Herdit, you will receive your very own hPhone, provided free-of-charge from the government! This phone comes with unlimited access to the internet as well as 500 gigabytes of storage for all your personal data. Herdit will use your hPhone to collect data for the sole purpose of adjusting your karmic score, colloquially known by the population as "karma". We use end-to-end encryption to keep all your data safe and secure on our servers.
|
|
||||||
|
|
||||||
In addition to your hPhone, your karmic score can also be modified from interactions in public spaces via security footage and others' hPhones, too! When you interact with others, your topic of discussion, mood, tone, and speaking style all are put through sophisticated technologies to ensure that your karmic score is as accurate as possible.
|
|
||||||
|
|
||||||
Your karmic score is very important to your life in Herdit. It is visible to every citizen in the tribe, and higher scores indicate that you are a better person in society! Herdit encourages competition for the highest possible karmic score, so engage in meaningful and factual discussion, work hard, and aim to help others raise their karmic scores, too! When you have a high karmic score, you are eligible for discounts, early access to public services, and other rewards!
|
|
||||||
|
|
||||||
Unfortunately, lower karmic scores may pose risks to a happy life in Herdit. Scores may be lowered as a result of an impolite demeanor, a lack of contribution to society, and the discussion of improper topics. If your score is too low, you may be required to head to the nearest Re-Education Centre so we can help you raise it back up to an acceptable level.
|
|
||||||
|
|
||||||
Thank you for considering Herdit citizenship, and we hope you strive to become the best person you can be!
|
|
@ -1,58 +0,0 @@
|
|||||||
---
|
|
||||||
title: Save File
|
|
||||||
date: 2020-07-22
|
|
||||||
tags:
|
|
||||||
- unstagnation
|
|
||||||
- literature
|
|
||||||
---
|
|
||||||
|
|
||||||
The sun shone brightly through an open window and its rays bounced happily around Peanut's house before slamming into his eyes.
|
|
||||||
<!-- excerpt -->
|
|
||||||
|
|
||||||
"Argh!" Peanut shut his eyes, holding back a few tears. "Stupid sun… Let me play my games!"
|
|
||||||
|
|
||||||
The sun no longer shone through the open window, its rays instead slamming into the thick curtains draped over the opening. Darkness reigned in the room for only a moment before electric lights illuminated a sleek-looking desktop computer setup. Sitting on a glossy wooden table, three large monitors displayed a video, collage of applications, and the desktop wallpaper, respectively.
|
|
||||||
|
|
||||||
Peanut blinked a few times to get the black spots out of his eyes, reclining all the way back in his gaming chair. It took a minute, but eventually he had dabbed all his salty eye fluids away. "Now, how do I install this…" he said, going back to clicking through his folder and files. "Maybe if I get rid of this and try again?"
|
|
||||||
|
|
||||||
*Are you sure you want to permanently delete this folder?*
|
|
||||||
|
|
||||||
"Yeah, I'm sure. What's in a NAND anyway? Just some system archives, right?"
|
|
||||||
|
|
||||||
*Folder deleted.*
|
|
||||||
|
|
||||||
"All I have to do is get the new dumped data in, and…" Peanut rubbed his hands together while he waited for the file copy operation to complete. "Let's try it!"
|
|
||||||
|
|
||||||
*System dump not found. Load failed.*
|
|
||||||
|
|
||||||
"Aw, man! What do you want me to do, junos? I've deleted and reinstalled and tried everything!" said Peanut, grumbling as he eventually closed all of his windows, pulling up junos again to play another game. "You win, for now. Rutadex it is, I guess."
|
|
||||||
|
|
||||||
*Please select your language.*
|
|
||||||
|
|
||||||
Peanut blinked. Then again. He squinted at the screen, hoping against hope that his heart wouldn't plummet any further as his brain asked his eyes to double-check that they had read the on-screen text correctly.
|
|
||||||
|
|
||||||
His save data, the save in which he had spent dozens of hours to grind and upgrade his character to the best character it could possibly be—all completely gone. As soon as his mind finally caught up to the present and fully, completely understood what had happened, he reeled back, shocked.
|
|
||||||
|
|
||||||
"Wha—How—what—when—h-how did this happen? No, that's impossible!" A thought occurred to him. "junos's save data is kept in the *NAND?* Oh, no… It might be salvageable!"
|
|
||||||
|
|
||||||
Typing furiously on his keyboard, he opened up terminal window after terminal window, launching all the data recovery tools he had.
|
|
||||||
|
|
||||||
*File not found.*
|
|
||||||
|
|
||||||
*File does not exist.*
|
|
||||||
|
|
||||||
*Error: Not found.*
|
|
||||||
|
|
||||||
*File not found: Check the path?*
|
|
||||||
|
|
||||||
*Space reallocated.*
|
|
||||||
|
|
||||||
As negative after negative flashed by on the screen, he was about to curl up into a ball and cry for days until one last program chirped a notification at him.
|
|
||||||
|
|
||||||
*File 00 found. Recover?*
|
|
||||||
|
|
||||||
"*YES! YES!* Oh, give me my gummies back, thank you testdisk I love you!" Gleefully, Peanut took the recovered file and plopped it into Rutadex's save directory as junos loaded in the background. "Still better check it, just in case…"
|
|
||||||
|
|
||||||
*Save data corrupted. Deleting and resetting…*
|
|
||||||
|
|
||||||
Wordlessly, Peanut stood up, gently pushed his chair away, and then went up to his room, where he lay down and cried himself to sleep.
|
|
@ -1,18 +0,0 @@
|
|||||||
---
|
|
||||||
title: Slush
|
|
||||||
date: 2020-07-29
|
|
||||||
tags:
|
|
||||||
- unstagnation
|
|
||||||
- literature
|
|
||||||
---
|
|
||||||
I poked at the green slush dripping down the table with a metal rod. "What…is this? It looks pretty tasty."
|
|
||||||
<!-- excerpt -->
|
|
||||||
Dr. Brown grinned maniacally. "Why, it's my patented magic alien slush, of course! What else *could* it be?"
|
|
||||||
|
|
||||||
The metal rod was instantly flung into the nearest hazardous waste disposal unit. "Huh. I thought it was a lime-flavoured snow cone. It looks pretty good, you know…"
|
|
||||||
|
|
||||||
The doctor waved a finger at me, shaking his head. "The mystic slush is not for human consumption, my good man. It is the key to solving all the world's problems! It contains power you would not believe! We shall conquer the world with the slush—well, maybe not conquer, but we will save it, my friend!"
|
|
||||||
|
|
||||||
I looked oddly at him. "All I hear are buzzwords. What does it actually *do?"*
|
|
||||||
|
|
||||||
Dr. Brown cleared his throat, gesturing majestically. "My slush will rule the planet—with its amazing power—the power of placebo!"
|
|
@ -1,43 +0,0 @@
|
|||||||
---
|
|
||||||
title: Student Over Teacher
|
|
||||||
date: 2021-06-13
|
|
||||||
tags:
|
|
||||||
- unstagnation
|
|
||||||
- literature
|
|
||||||
---
|
|
||||||
|
|
||||||
"You have failed our people for the last time, Rooster." Yammy's declaration was intensified by the kneeling man with his hands tied before him. Briefly, a hole parted in the grey cloud cover, allowing a single sunbeam to illuminate Yammy's face as he looked out at the crowd assembled in front of him. "Out with the old and in with the new, as they say," he chuckled.
|
|
||||||
|
|
||||||
<!-- excerpt -->
|
|
||||||
|
|
||||||
"Just you wait," spat Rooster, an angry and determined expression on his face disregarding the foot pressing the side of his head into the wooden stage. "You'll run down the village just like every other leader before you. Mark my words, you'll be overthrown one day — and that is when I shall return from my exile and restore balance."
|
|
||||||
|
|
||||||
Yammy twisted his foot harder into Rooster's head, but threw his own back and laughed. "Exile? You think you'll be going into exile? Oh, no, my old master, don't you remember what you taught me? 'The best way to deal with your opponents is to not have any opponents at all,' you said. That's what this," he said, flicking a hoop made of rope suspended in the air by a wooden beam, "is for. You will not live long enough to see my permanent rule." Yammy brought his foot off of Rooster's head and tapped it twice against the ground. "Bring forth the steps!"
|
|
||||||
|
|
||||||
Two men in heavy armour parted the crowd by hoisting a set of light-looking wooden stairs up the stage.
|
|
||||||
|
|
||||||
"You bastard! I would never — in my twenty years as head of this village, I have never executed a single person!"
|
|
||||||
|
|
||||||
"Maybe you should have, to get rid of your opponents," Yammy winked. "Take him up!"
|
|
||||||
|
|
||||||
There was little struggle as the two guards roughly yanked Rooster to his feet and pushed him up the steps to the gallows. "You'll never get away with this!" His attempt to turn his head back to yell at Yammy was ruined by a guard shoving his head into the rope hoop.
|
|
||||||
|
|
||||||
"Whenever you're ready." One guard motioned to the other, who nodded and took a step back. Rooster closed his eyes, ready for the inevitable.
|
|
||||||
|
|
||||||
A *swish* above his head and gasps from the crowd immediately let him know something was off. A sweaty hand tugged on his own, and Rooster turned to see a stout young boy dragging him along as he stumbled out of the iron armour set, which was cut in two. "Master, let's go!" he said, face shiny and flushed.
|
|
||||||
|
|
||||||
Yammy was furious. "Traitor! Apprehend them both at once!"
|
|
||||||
|
|
||||||
Rooster didn't hesitate and threw the rope around his neck behind him. Before the second guard could impale them both with his deadly-looking spear, he sped up ahead of the boy off the stage and onto the dirt road, looking back occasionally to make sure he could keep up. "Move, boy, move!" he yelled as he felt the tension on his hand increase. "You should have ridden those pounds years ago!"
|
|
||||||
|
|
||||||
The *clank clank clank* of iron on ground became quieter and quieter as the duo put more distance between them and their pursuers until they reached the edge of a thick line of trees. "Do not let go of my hand, boy!"
|
|
||||||
|
|
||||||
"Stop!" Yammy ordered as they reached the tree line shortly after, holding the guard behind with an outstretched arm. "We will never catch them if we chase after them in the Forest of Git. But no matter. The forest will take care of them for us."
|
|
||||||
|
|
||||||
---
|
|
||||||
|
|
||||||
"Foolish boy. Napbot, that was incredibly reckless of you and not at all necessary."
|
|
||||||
|
|
||||||
The boy sat with his back against a tree, heaving. "I…couldn't…let you…go like that, master. Yammy…what he's doing is…not right. And I would have been executed soon after because of you."
|
|
||||||
|
|
||||||
Rooster sighed. "That…is true," he admitted. "I must apologise for associating with you. From this day on, we will live as fugitives off of the Forest of Git — until we can reclaim what is rightfully ours."
|
|
@ -1,27 +0,0 @@
|
|||||||
---
|
|
||||||
title: The Leeway of Leeco
|
|
||||||
date: 2020-06-14
|
|
||||||
tags:
|
|
||||||
- barin
|
|
||||||
- unstagnation
|
|
||||||
- literature
|
|
||||||
---
|
|
||||||
|
|
||||||
I confidently strode up to my teacher, a fistful of papers in my left hand. Mrs. Lowshi smiled as I approached. “Good morning, Bobby. Are you here to hand in yesterday’s homework?”
|
|
||||||
<!-- excerpt -->
|
|
||||||
|
|
||||||
Well, I had been playing video games all night. What use was homework anyway? I put on as convincing of a disappointed face as I could. “I’m so sorry, Mrs. Lowshi, but I couldn’t do it. Every time I tried, my dog ate it! Here’s all I could salvage from his paper-thirsty mouth…” The fistful of shredded paper was dropped on Mrs. Lowshi’s desk, who brought hands up to her mouth in horror.
|
|
||||||
|
|
||||||
“Goodness! Why, I can’t imagine how many times you had to redo the work!”
|
|
||||||
|
|
||||||
I nodded as despairingly as I could manage, but a large grin had broken out inside me. Of course she fell for it! Every teacher in Leeco was trained so that they were more “open and tolerant” after the whole Kora incident. It was a great change, if you ask me. School is just a free babysitting program for parents to drop off their children before they go to work. Completely useless.
|
|
||||||
|
|
||||||
I squinted as hard as I could to get a tear to fall out of my eye. The more dramatic, the better. “I did, Mrs. Lowshi! I did! You have to believe me! I—” I paused for dramatic effect, and my teacher took the opportunity to bring me around to her side of the desk.
|
|
||||||
|
|
||||||
“There, there,” she said as she patted me on the back in a soothing tone. “Don’t worry about the work, now. I’m not going to make you do it again. I’m sure you know the content by heart already. Would you like a tissue before we begin the test?”
|
|
||||||
|
|
||||||
I shook my head, then wordlessly headed back to my table. As I brushed past my friend’s desk, I tapped it twice. He got the message and headed up to Mrs. Lowshi’s desk, who looked at him concernedly. “Joey, what’s wrong?”
|
|
||||||
|
|
||||||
“Mrs. Lowshi! You have to help me! We can’t take the test today! I was studying with my friends last night, and none of us could understand anything!”
|
|
||||||
|
|
||||||
Impressive. He managed to shed at least five tears there. As I sat down in front of my desk, my teacher’s voice drifted up from the front of the room. “Oh, it’s all right, Joey. We won’t make you take the test. We’ll spend a few more days reviewing before we move on to the next unit. Is that okay?”
|
|
@ -1,36 +0,0 @@
|
|||||||
---
|
|
||||||
title: The Second Front
|
|
||||||
date: 2021-06-16
|
|
||||||
tags:
|
|
||||||
- barin
|
|
||||||
- unstagnation
|
|
||||||
- literature
|
|
||||||
---
|
|
||||||
|
|
||||||
Maquiate Todofuken Administration Centre\
|
|
||||||
1 Todofuken Road\
|
|
||||||
Maquiate, Asvyn
|
|
||||||
|
|
||||||
<!-- excerpt -->
|
|
||||||
|
|
||||||
Hina Asvyn\
|
|
||||||
Empress\
|
|
||||||
1 Kansei Road\
|
|
||||||
Emina, Asvyn
|
|
||||||
|
|
||||||
Empress,
|
|
||||||
|
|
||||||
I am pleased to report that the negotiations with the recently Enigma-seceded region of Maquiate have concluded very much in the favour of the Empire. The region has pledged allegiance to Emina and are currently assisting in driving out rebellious forces with their sizable military. It is predicted that the Empire will be unified within several days with the reinforcements from Maquiate.
|
|
||||||
|
|
||||||
Additionally, the Favonius organisation has put forth a proposal: they are willing to cease all activities in the Empire should we offer them a free trade agreement. The Principal of Intelligence notes that the organisation has heavy ties to the Preton Alliance, which could potentially open up the Empire up to exploitation in the future. It is advised that the current campaign remain unchanged to remove the threat of Favonius by force, as there is no advantage to diplomacy in the current situation.
|
|
||||||
|
|
||||||
The regions of Aineiru, Shiganshina, Kanokari, and Shuchiin have been secured with minimal losses. Pleiades has pledged full support to the Empire as well and has ejected its Favonius members. At this point in time, most of our efforts are targeting the remaining Romcom and Tonikawa. It is advised that Romcom focused on due to its dominance over Tonikawa. Should Romcom fall, Tonikawa will fall as well.
|
|
||||||
|
|
||||||
The Imperial Proclamation with reference to the Enigma withdrawal has resulted in reduced morale across Empire-supporting territories, many of whom had withdrawn from the Enigma Alliance after the Empire was the first to do so. If I may, I must comment that this is a peculiar action to take — after years of negotiations and stalls, the Empire rejoins the Enigma Alliance. It has not quite caused upheaval throughout the Empire yet, but there are already troubling signs during these unstable times.
|
|
||||||
|
|
||||||
It is therefore my recommendation that efforts be focused on strengthening the Interior before managing External affairs.
|
|
||||||
|
|
||||||
Yours,\
|
|
||||||
Kuvira Smith\
|
|
||||||
Principal of the Interior\
|
|
||||||
14 June 2021
|
|
@ -1,42 +0,0 @@
|
|||||||
---
|
|
||||||
title: Uprising
|
|
||||||
date: 2021-05-24
|
|
||||||
tags:
|
|
||||||
- barin
|
|
||||||
- unstagnation
|
|
||||||
- literature
|
|
||||||
---
|
|
||||||
The Sinamaria Rose\
|
|
||||||
12 Dedication Road\
|
|
||||||
Emina, Asvyn
|
|
||||||
|
|
||||||
<!-- excerpt -->
|
|
||||||
|
|
||||||
Hina Asvyn\
|
|
||||||
Empress\
|
|
||||||
1 Kansei Road\
|
|
||||||
Emina, Asvyn
|
|
||||||
|
|
||||||
Empress,
|
|
||||||
|
|
||||||
It has been observed that some regions in the state have been discouraged by the pace of the withdrawal from the Enigma Alliance. There is a threat that is provoking citizens and sparking unrest in areas of greatest tension, pressuring them to conduct trade with Preton in breach of Section 1 of the Saiyu Agreement, which remains in force until withdrawal negotiations are concluded and signed. The group calls themselves "Favonius". A status report of the group's activities and their impacts on each region are listed below.
|
|
||||||
|
|
||||||
- Emina: The capital city remains fully under control of the Empire. Citizens living in Emina are generally strongly opposed to the activities of this new group. No action is recommended for this region.
|
|
||||||
- Kolalta: Much like Emina, Kolalta is under little danger as the region detests the Preton Alliance. No action is recommended for this region.
|
|
||||||
- Pleiades: The Principal for Intelligence has determined that the origins of Favonius originate in Pleiades. The state of affairs in this region is more normal than many of the other regions that they have spread to.
|
|
||||||
- Shiganshina: The peasant majority in Shiganshina have been easily convinced by Favonius and are currently refusing to follow orders from the Empire. It is advised that the military be applied to reassert control over the region.
|
|
||||||
- Kanokari: Favonius has gained full control over the local government in Kanokari. All demands and ultimata have been ignored. It is advised that the military be applied to reassert control over the region.
|
|
||||||
- Keion: For whatever reason, Favonius has not advanced in this region at all, and Keion has not acknowledged Favonius, either. It is advised that surveillance be increased in this region to determine the cause behind the anomalous behaviour.
|
|
||||||
- Shuchiin: The semi-autonomous region believes that allying with Favonius and conducting trade with Preton will further line their pockets. Favonius supporters have secured the majority of the seats in the legislative council and they are unreceptive to our demands. It is advised that the military be applied to reassert control over the region.
|
|
||||||
- Romcom: Romcom has fallen completely to Favonius and they are currently waging war against the neighbouring regions of Ruvinheigen, Tonikawa, and Musani in an effort to bring them under control. It is advised that reinforcements be sent to those regions to prevent the fall of more regions to Favonius.
|
|
||||||
- Musani: Musani is currently completely stable even against the Romconian attack. Resistance against Favonius is very strong in all levels of authority. No action is recommended for this region.
|
|
||||||
- Tonikawa: Nearly half of Tonikawa has been captured by Romcom and the situation is critical. It is strongly advised that the military be more heavily applied to this region.
|
|
||||||
- Ruvinheigen: This semi-autonomous region is currently stable but there are rumours behind the scenes about a possible Favonius uprising. It is recommended that surveillance be sharply increased in this region to monitor the situation as it changes.
|
|
||||||
- Aineiru: Favonius has just started taking root in this region and it is causing a small but growing impact on government control. It is advised that specialised agents be used to eliminate problematic persons to prevent further growth of confusion.
|
|
||||||
|
|
||||||
The situation is quite dire in many regions of the Empire, Empress, and I hope that you will allocate us the troops we need to shut down this attack on our state.
|
|
||||||
|
|
||||||
Yours,\
|
|
||||||
Kuvira Smith\
|
|
||||||
Principal for Homeland Security\
|
|
||||||
24 May 2021
|
|
@ -1,42 +0,0 @@
|
|||||||
---
|
|
||||||
title: Wet Hair
|
|
||||||
date: 2020-06-26
|
|
||||||
tags:
|
|
||||||
- unstagnation
|
|
||||||
- literature
|
|
||||||
---
|
|
||||||
|
|
||||||
*Splash!*
|
|
||||||
|
|
||||||
A flying projectile hits the back of Cloche's head. It explodes, soaking her whole body as she lurches forward from the impact, which is not quite strong enough to cause any lasting pain. She pauses for a moment, then slowly turns around to face the perpetrator.
|
|
||||||
<!-- excerpt -->
|
|
||||||
|
|
||||||
Garson is smiling obliviously at her inner demons, and laughs at her misfortune.
|
|
||||||
|
|
||||||
"Ha! Got you, Cloche!"
|
|
||||||
|
|
||||||
Cloche runs her fingers through her now-wet hair, which stretches down to her waist. Her voice becomes quiet. "That was you?"
|
|
||||||
|
|
||||||
"Man, I thought I'd never be able to sneak up on you! I bet you didn't hear a thing, did you!"
|
|
||||||
|
|
||||||
Cloche stays silent for a few seconds.
|
|
||||||
|
|
||||||
"Uh, Cloche? Are you okay? You seem…a little bit more annoyed than I thought you were…"
|
|
||||||
|
|
||||||
Finally, she can take no more of this *boy's* nonsense and explodes.
|
|
||||||
|
|
||||||
"You *inconsiderate* *scoundrel!"* she yells, shaking. "Do you *realise* what you've done?"
|
|
||||||
|
|
||||||
Garson looks to be taken aback. "What—"
|
|
||||||
|
|
||||||
"You *wet* my *hair!* It takes *hours* upon *hours* for this mass of keratin to dry out properly, and does it *look* like we're near a hair dryer anywhere? I trusted you, Garson! I trusted you! And you had the sheer *nerve* to go behind my back and *soak* me! I should take you to court for assault!"
|
|
||||||
|
|
||||||
"I'm sor—"
|
|
||||||
|
|
||||||
" 'Sorry' isn't going to cut it here, Garson!" Cloche steps closer to him, glare intensifying. "What you have done to me is completely unacceptable and condones *cruel and unusual punishment*, but since I'm a forgiving girl, I'll let you off with one last warning. Do I make myself clear?" She hisses the last sentence right up to the pale-faced Garson.
|
|
||||||
|
|
||||||
"Yes, ma'am!" Garson squeaks.
|
|
||||||
|
|
||||||
Cloche spins around, causing her waterlogged hair to follow after, slamming into Garson's face like a sledgehammer. He stumbles backward, disoriented.
|
|
||||||
|
|
||||||
"Owie…" Garson mutters. "Are those rockets, mommy?"
|
|
@ -1,42 +0,0 @@
|
|||||||
---
|
|
||||||
layout: typical
|
|
||||||
title: Services
|
|
||||||
---
|
|
||||||
|
|
||||||
**[Server uptime status](https://uptime.eggworld.tk)**
|
|
||||||
|
|
||||||
This site is generated by the static site generator [Eleventy](https://www.11ty.dev) with templates and Markdown — because really, writing HTML by hand is tedious and I don't know why I ever tried — and its source is available [here](https://git.eggworld.tk/eggy/public). The theme this site uses is taken from [here.](https://github.com/kohrongying/11ty-blog-starter)
|
|
||||||
|
|
||||||
The following services are publicly running on this server ("asvyn"):
|
|
||||||
|
|
||||||
## [Gitea](https://git.eggworld.tk)
|
|
||||||
|
|
||||||
Gitea hosts the Eifueo project as well as a few other items that I deem not worthy to go on GitHub.
|
|
||||||
|
|
||||||
## [Eifueo](https://eifueo.eggworld.tk)
|
|
||||||
|
|
||||||
Generated by [MkDocs](https://mkdocs.org), this site aims to collate my notes and I hope others might find it helpful.
|
|
||||||
|
|
||||||
## [Primoprod](https://primoprod.eggworld.tk)
|
|
||||||
|
|
||||||
This is a side project for fun that attempts to recreate a video game UI as much as possible using standard web technologies.
|
|
||||||
|
|
||||||
## [Calibre](https://calibre.eggworld.tk)
|
|
||||||
|
|
||||||
As an avid reader of…"user-generated content", I store a database of stories here and sync them with my Kobo.
|
|
||||||
|
|
||||||
---
|
|
||||||
|
|
||||||
The following services are publicly running on the more powerful secondary server ("panquia"):
|
|
||||||
|
|
||||||
## [Plex](https://plex.eggworld.tk)
|
|
||||||
|
|
||||||
This was beyond stupid and took far too much time to get working behind nginx. I'm currently working to replace it with Jellyfin as it becomes more mature.
|
|
||||||
|
|
||||||
## [Jellyfin](https://jellyfin.eggworld.tk)
|
|
||||||
|
|
||||||
Jellyfin has improved significantly in the past two years since I last looked at it. There are only a couple of roadblocks before full adoption and replacing Plex.
|
|
||||||
|
|
||||||
## Minecraft
|
|
||||||
|
|
||||||
The domain constantly changes and the server is whitelisted, so don't bother looking.
|
|
@ -1,16 +0,0 @@
|
|||||||
---
|
|
||||||
permalink: /sitemap.xml
|
|
||||||
eleventyExcludeFromCollections: true
|
|
||||||
---
|
|
||||||
<?xml version="1.0" encoding="utf-8"?>
|
|
||||||
<urlset xmlns="http://www.sitemaps.org/schemas/sitemap/0.9">
|
|
||||||
{% for page in collections.all %}
|
|
||||||
{% if not page.data.draft %}
|
|
||||||
<url>
|
|
||||||
<loc>{{ site.url }}{{ page.url | url }}</loc>
|
|
||||||
<lastmod>{{ page.date }}</lastmod>
|
|
||||||
<changefreq>{{page.data.changeFreq}}</changefreq>
|
|
||||||
</url>
|
|
||||||
{% endif %}
|
|
||||||
{% endfor %}
|
|
||||||
</urlset>
|
|
@ -1,22 +0,0 @@
|
|||||||
---
|
|
||||||
layout: default
|
|
||||||
pagination:
|
|
||||||
data: collections
|
|
||||||
size: 1
|
|
||||||
alias: tag
|
|
||||||
permalink: /tags/{{ tag }}/
|
|
||||||
eleventyComputed:
|
|
||||||
title: "{{ tag }}"
|
|
||||||
---
|
|
||||||
|
|
||||||
{% for post in collections[tag] reversed %}
|
|
||||||
<div class="py-4 sm:py-4">
|
|
||||||
<p>
|
|
||||||
<span class="text-2xl sm:text-2xl font-bold hover:underline"><a href="{{ post.url }}">{{ post.data.title }}</a></span>
|
|
||||||
</p>
|
|
||||||
<em>{{ post.date | realDate | date: "%Y-%m-%d" }}</em>
|
|
||||||
<p class="mt-4">{{ post.data.post_excerpt }}...
|
|
||||||
<span class="hover:underline text-indigo-500"><a href="{{ post.url }}">Read More</a></span>
|
|
||||||
</p>
|
|
||||||
</div>
|
|
||||||
{% endfor %}
|
|